
编写一个shell脚本,读取指定目录下的所有可读的普通文件,将这些文件中的内容
1个回答
关注
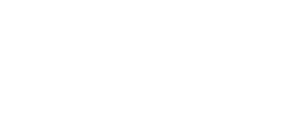
展开全部
# 读取指定目录下的所有可读的普通文件,将这些文件中的内容依次写入该目录下的total.txt文件中
if [ $# -ne 1 ]; then
echo "Usage: $0 directory"
exit 1
fi
dir=$1
if [ ! -d "$dir" ]; then
echo "$dir is not a directory"
exit 1
fi
if [ ! -w "$dir" ]; then
echo "$dir is not writable"
exit 1
fi
total_file="$dir/total.txt"
if [ -e "$total_file" ]; then
echo "total.txt already exists, please remove it first"
exit 1
fi
touch "$total_file"
for file in "$dir"/*; do
if [ -f "$file" ] && [ -r "$file" ]; then
cat "$file" >> "$total_file"
fi
done
echo "Done"
咨询记录 · 回答于2024-01-03
编写一个shell脚本,读取指定目录下的所有可读的普通文件,将这些文件中的内容
编写一个shell脚本,读取指定目录下的所有可读的普通文件,将这些文件中的内容依次写入该目录下的total.txt文件中,指定目录通过位置参数传入脚本。
# 读取指定目录下的所有可读的普通文件,将这些文件中的内容依次写入该目录下的total.txt文件中
if [ $# -ne 1 ]; then
echo "Usage: $0 directory"
exit 1
fi
dir=$1
if [ ! -d "$dir" ]; then
echo "$dir is not a directory"
exit 1
fi
if [ ! -w "$dir" ]; then
echo "$dir is not writable"
exit 1
fi
total_file="$dir/total.txt"
if [ -e "$total_file" ]; then
echo "total.txt already exists, please remove it first"
exit 1
fi
touch "$total_file"
for file in "$dir"/*; do
if [ -f "$file" ] && [ -r "$file" ]; then
cat "$file" >> "$total_file"
fi
done
echo "Done"
以下是一个示例脚本,可以实现读取指定目录下的所有可读的普通文件,将这些文件中的内容依次写入该目录下的total.txt文件中:
#!/bin/bash
# 检查是否传入了目录参数
if [ -z "$1" ]; then
echo "Usage: $0 directory"
exit 1
fi
# 检查指定目录是否存在
if [ ! -d "$1" ]; then
echo "Error: directory $1 does not exist"
exit 1
fi
# 检查total.txt文件是否存在,如果不存在则创建
if [ ! -f "$1/total.txt" ]; then
touch "$1/total.txt"
fi
# 遍历目录下的所有可读的普通文件
for file in $(find "$1" -type f -readable); do
# 将文件内容追加到total.txt文件中
cat "$file" >> "$1/total.txt"
done
echo "Done"
# 使用方法:将上述脚本保存为一个文件,例如merge_files.sh,然后在终端中执行以下命令:
# $ chmod +x merge_files.sh # 添加执行权限
# $ ./merge_files.sh /path/to/directory # 替换成实际的目录路径
# 执行完毕后,指定目录下的所有可读的普通文件的内容将被依次写入该目录下的total.txt文件中。
他说then附近有语法错误哎
亲,这个不会有错的额
以下是一个可能的解决方案:
#!/bin/bash
if [ $# -ne 1 ]; then
echo "Usage: $0 directory"
exit 1
fi
dir=$1
total_file="$dir/total.txt"
if [ -e "$total_file" ]; then
echo "Warning: $total_file already exists, will be overwritten."
fi
echo -n > "$total_file" # 清空total.txt文件
for file in "$dir"/*; do
if [ -f "$file" ] && [ -r "$file" ]; then
cat "$file" >> "$total_file"
fi
done
echo "All readable files in $dir have been concatenated to $total_file."
# 说明:
# 这个脚本首先检查是否有一个参数传递给它,如果没有,则打印用法信息并退出。
# 然后,它设置一些变量,包括total.txt的路径,然后检查该文件是否已经存在。如果它存在,它会发出警告并将其覆盖。
# 然后,它清空total.txt文件,以便我们可以将所有文件的内容写入其中。
# 接下来,它使用一个循环来遍历指定目录下的所有文件。如果文件是一个普通文件并且可读,则将其内容附加到total.txt文件中。
# 最后,它打印一条消息,指示所有可读文件已经被连接到total.txt文件中。
# 要使用此脚本,请将其保存为concat.sh(或其他名称),然后将其设置为可执行文件:chmod +x concat.sh
# 然后,您可以通过传递目录路径作为参数来运行它:./concat.sh /path/to/directory
# 请注意,此脚本不会递归地遍历子目录中的文件。如果您想要这样做,您可以将循环改为使用find命令。