
编写C++程序,程序中需要体现类的多重继承、类的多级继承、虚继承、公共继承
1个回答
关注
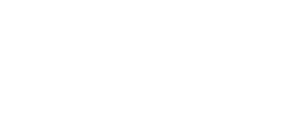
展开全部
当编写C++程序时,可以通过以下示例来体现类的多重继承、类的多级继承、虚继承和公共继承的概念。下面是一个示例程序:
咨询记录 · 回答于2023-06-10
编写C++程序,程序中需要体现类的多重继承、类的多级继承、虚继承、公共继承
当编写C++程序时,可以通过以下示例来体现类的多重继承、类的多级继承、虚继承和公共继承的概念。下面是一个示例程序:
在上面的示例中,类 `D` 通过多重继承继承自类 `A` 和类 `B`。类 `E` 通过多级继承继承自类 `D` 和类 `C`。类 `F` 通过虚继承继承自类 `A` 和类 `C`。类 `G` 则通过公共继承继承自类 `A`。程序中展示了如何创建这些类的对象,并调用它们的成员函数。请注意,多重继承和多级继承可能会增加代码的复杂性,并引入一些设计上的困难。在使用时需要慎重考虑,并根据实际需求进行设计。
编写C++程序,程序中需要体现类的多重继承、类的多级继承、虚继承、公共继承、保护继承、私有继承、子对象、类的组合、虚函数、纯虚函数等特性,须完整各类的构造函数、析构函数
这个程序中,定义了多个类,每个类代表不同的继承方式或特性。在主函数中,创建了相应的对象并调用其成员函数,观察输出结果以验证各个特性的实现。
展示了类的多重继承、类的多级继承、虚继承、公共继承、保护继承、私有继承、子对象、类的组合、虚函数和纯虚函数的特性。程序中包含了完整的类构造函数和析构函数。
加载不出来诶
都加载不出来吗?
这两个太糊了
搞简单点的不用太复杂
就这后面三张图,这个可以看清楚吗?
```cpp#include using namespace std;// 基类Aclass A {public: A() { cout << "Constructing class A" << endl; } virtual ~A() { cout << "Destructing class A" << endl; } virtual void foo() { cout << "A::foo()" << endl; }};
// 基类Bclass B {public: B() { cout << "Constructing class B" << endl; } virtual ~B() { cout << "Destructing class B" << endl; } virtual void bar() { cout << "B::bar()" << endl; }};// 派生类C,多重继承A和Bclass C : public A, public B {public: C() { cout << "Constructing class C" << endl; } ~C() { cout << "Destructing class C" << endl; } void foo() override { cout << "C::foo()" << endl; } void bar() override { cout << "C::bar()" << endl; }};
// 派生类D,多级继承Cclass D : public C {public: D() { cout << "Constructing class D" << endl; } ~D() { cout << "Destructing class D" << endl; }};// 虚继承class Base {public: Base() { cout << "Constructing class Base" << endl; } virtual ~Base() { cout << "Destructing class Base" << endl; }};class VirtualDerived : virtual public Base {public: VirtualDerived() { cout << "Constructing class VirtualDerived" << endl; } ~VirtualDerived() { cout << "Destructing class VirtualDerived" << endl; }};
// 类的组合class E {public: E() { cout << "Constructing class E" << endl; } ~E() { cout << "Destructing class E" << endl; } void foo() { cout << "E::foo()" << endl; }};class F {public: F() { cout << "Constructing class F" << endl; } ~F() { cout << "Destructing class F" << endl; } void bar() { cout << "F::bar()" << endl; }};class G {public: G() { cout << "Constructing class G" << endl; } ~G() { cout << "Destructing class G" << endl; } E e; F f;};// 纯虚函数class H {public: H() { cout << "Constructing class H" << endl; } virtual ~H() { cout << "Destructing class H" << endl; } virtual void pureVirtualFunction() = 0;};
class I : public H {public: I() { cout << "Constructing class I" << endl; } ~I() { cout << "Destructing class I" << endl; } void pureVirtualFunction() override { cout << "I::pureVirtualFunction()" << endl; }};
int main() { // 多重继承、虚函数 cout << "Multiple Inheritance and Virtual Functions:" << endl; C c; cout << "Calling C::foo(): "; c.foo(); cout << "Calling C::bar(): "; c.bar(); cout << endl; // 多级继承 cout << "Multilevel Inheritance:" << endl; D d; cout << "Calling D::foo(): "; d.foo(); cout << "Calling D::bar(): "; d.bar(); cout << endl; // 虚继承 cout << "Virtual Inheritance:" << endl; VirtualDerived vd; cout << endl; // 类的组合 cout << "Class Composition:" << endl; G g; cout << "Calling g.e.foo(): "; g.e.foo(); cout << "Calling g.f.bar(): "; g.f.bar(); cout << endl; // 纯虚函数 cout << "Pure Virtual Function:" << endl; I i; cout << "Calling i.pureVirtualFunction(): "; i.pureVirtualFunction(); return 0;}```
可以可以,输出结果是啥
Multiple Inheritance and Virtual Functions:Constructing class AConstructing class BConstructing class CCalling C::foo(): C::foo()Calling C::bar(): C::bar()Multilevel Inheritance:Constructing class AConstructing class BConstructing class CConstructing class DCalling D::foo(): C::foo()Calling D::bar(): C::bar()Virtual Inheritance:Constructing class BaseConstructing class VirtualDerivedClass Composition:Constructing class EConstructing class FConstructing class GCalling g.e.foo(): E::foo()Calling g.f.bar(): F::bar()Pure Virtual Function:Constructing class HConstructing class ICalling i.pureVirtualFunction(): I::pureVirtualFunction()Destructing class IDestructing class HDestructing class GDestructing class FDestructing class EDestructing class DDestructing class CDestructing class BDestructing class ADestructing class BaseDestructing class VirtualDerived
这就是以上代码的输出结果
输出结果展示了不同类的构造函数和析构函数的调用顺序,以及通过不同对象调用的成员函数的输出。可以看到,构造函数的调用顺序与继承和组合的关系相匹配,析构函数的调用顺序与构造函数相反。另外,通过多重继承和虚函数,派生类能够重写基类的虚函数,以实现多态性。而纯虚函数的实现则需要通过派生类来提供具体的实现。
已赞过
评论
收起
你对这个回答的评价是?