
麻烦简述cookie的设置和获取过程。谢谢
1个回答
2016-02-29 · 知道合伙人软件行家
关注
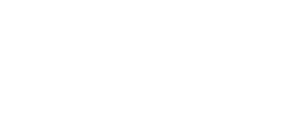
展开全部
1、设置请求消息上的 Cookie
为 HttpWebRequest 的 HttpWebRequest.CookieContainer 属性创建一个 System.Net.CookieContainer 对象。
C#
VB
request.CookieContainer = new CookieContainer();
2、使用 CookieContainer.Add 方法将 Cookie 对象添加到 HttpWebRequest.CookieContainer。
C#
VB
request.CookieContainer.Add(new Uri("http://api.search.live.net"), new Cookie("id", "1234"));
3、获取响应消息上的 Cookie
在请求上创建一个 System.Net.CookieContainer 以保存对响应发送的 Cookie 对象。 即使没有发送任何 Cookie 也必须执行此操作。
C#
VB
request.CookieContainer = new CookieContainer();
4、检索 HttpWebResponse 的 HttpWebResponse.Cookies 属性中的值。 在此示例中,将检索 Cookie 并将它们保存到独立存储中。
C#
VB
private void ReadCallback(IAsyncResult asynchronousResult) { HttpWebRequest request = (HttpWebRequest)asynchronousResult.AsyncState; HttpWebResponse response = (HttpWebResponse) request.EndGetResponse(asynchronousResult); using (IsolatedStorageFile isf = IsolatedStorageFile.GetUserStoreForSite()) { using (IsolatedStorageFileStream isfs = isf.OpenFile("CookieExCookies", FileMode.OpenOrCreate, FileAccess.Write)) { using (StreamWriter sw = new StreamWriter(isfs)) { foreach (Cookie cookieValue in response.Cookies) { sw.WriteLine("Cookie: " + cookieValue.ToString()); } sw.Close(); } } } }
示例
下面的示例演示如何创建 Web 请求并向该请求添加 Cookie。 此示例还演示如何从 Web 响应提取 Cookie,如何将 Cookie 写入独立存储中的文件以及从独立存储中进行读取。 运行此示例时,将在 TextBlock 控件中显示 System.Net.Cookie 值。
运行此示例
C#
VB
using System; using System.Collections.Generic; using System.Linq; using System.Net; using System.Windows; using System.Windows.Controls; using System.Windows.Documents; using System.Windows.Input; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Shapes; using System.Net.Browser; using System.IO; using System.Text; using System.IO.IsolatedStorage; namespace CookiesEx { public partial class MainPage : UserControl { public MainPage() { InitializeComponent(); } private void button1_Click(object sender, RoutedEventArgs e) { InitializeWebRequestClientStackForURI(); ReadFromIsolatedStorage(); } private void InitializeWebRequestClientStackForURI() { // Create the client WebRequest creator. IWebRequestCreate creator = WebRequestCreator.ClientHttp; // Register both http and https. WebRequest.RegisterPrefix("http://", creator); WebRequest.RegisterPrefix("https://", creator); // Create a HttpWebRequest. HttpWebRequest request = (HttpWebRequest) WebRequest.Create("http://api.search.live.net/clientaccesspolicy.xml"); //Create the cookie container and add a cookie. request.CookieContainer = new CookieContainer(); // This example shows manually adding a cookie, but you would most // likely read the cookies from isolated storage. request.CookieContainer.Add(new Uri("http://api.search.live.net"), new Cookie("id", "1234")); // Send the request. request.BeginGetResponse(new AsyncCallback(ReadCallback), request); } // Get the response and write cookies to isolated storage. private void ReadCallback(IAsyncResult asynchronousResult) { HttpWebRequest request = (HttpWebRequest)asynchronousResult.AsyncState; HttpWebResponse response = (HttpWebResponse) request.EndGetResponse(asynchronousResult); using (IsolatedStorageFile isf = IsolatedStorageFile.GetUserStoreForSite()) { using (IsolatedStorageFileStream isfs = isf.OpenFile("CookieExCookies", FileMode.OpenOrCreate, FileAccess.Write)) { using (StreamWriter sw = new StreamWriter(isfs)) { foreach (Cookie cookieValue in response.Cookies) { sw.WriteLine("Cookie: " + cookieValue.ToString()); } sw.Close(); } } } } private void ReadFromIsolatedStorage() { using (IsolatedStorageFile isf = IsolatedStorageFile.GetUserStoreForSite()) { using (IsolatedStorageFileStream isfs = isf.OpenFile("CookieExCookies", FileMode.Open)) { using (StreamReader sr = new StreamReader(isfs)) { tb1.Text = sr.ReadToEnd(); sr.Close(); } } } } } }
为 HttpWebRequest 的 HttpWebRequest.CookieContainer 属性创建一个 System.Net.CookieContainer 对象。
C#
VB
request.CookieContainer = new CookieContainer();
2、使用 CookieContainer.Add 方法将 Cookie 对象添加到 HttpWebRequest.CookieContainer。
C#
VB
request.CookieContainer.Add(new Uri("http://api.search.live.net"), new Cookie("id", "1234"));
3、获取响应消息上的 Cookie
在请求上创建一个 System.Net.CookieContainer 以保存对响应发送的 Cookie 对象。 即使没有发送任何 Cookie 也必须执行此操作。
C#
VB
request.CookieContainer = new CookieContainer();
4、检索 HttpWebResponse 的 HttpWebResponse.Cookies 属性中的值。 在此示例中,将检索 Cookie 并将它们保存到独立存储中。
C#
VB
private void ReadCallback(IAsyncResult asynchronousResult) { HttpWebRequest request = (HttpWebRequest)asynchronousResult.AsyncState; HttpWebResponse response = (HttpWebResponse) request.EndGetResponse(asynchronousResult); using (IsolatedStorageFile isf = IsolatedStorageFile.GetUserStoreForSite()) { using (IsolatedStorageFileStream isfs = isf.OpenFile("CookieExCookies", FileMode.OpenOrCreate, FileAccess.Write)) { using (StreamWriter sw = new StreamWriter(isfs)) { foreach (Cookie cookieValue in response.Cookies) { sw.WriteLine("Cookie: " + cookieValue.ToString()); } sw.Close(); } } } }
示例
下面的示例演示如何创建 Web 请求并向该请求添加 Cookie。 此示例还演示如何从 Web 响应提取 Cookie,如何将 Cookie 写入独立存储中的文件以及从独立存储中进行读取。 运行此示例时,将在 TextBlock 控件中显示 System.Net.Cookie 值。
运行此示例
C#
VB
using System; using System.Collections.Generic; using System.Linq; using System.Net; using System.Windows; using System.Windows.Controls; using System.Windows.Documents; using System.Windows.Input; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Shapes; using System.Net.Browser; using System.IO; using System.Text; using System.IO.IsolatedStorage; namespace CookiesEx { public partial class MainPage : UserControl { public MainPage() { InitializeComponent(); } private void button1_Click(object sender, RoutedEventArgs e) { InitializeWebRequestClientStackForURI(); ReadFromIsolatedStorage(); } private void InitializeWebRequestClientStackForURI() { // Create the client WebRequest creator. IWebRequestCreate creator = WebRequestCreator.ClientHttp; // Register both http and https. WebRequest.RegisterPrefix("http://", creator); WebRequest.RegisterPrefix("https://", creator); // Create a HttpWebRequest. HttpWebRequest request = (HttpWebRequest) WebRequest.Create("http://api.search.live.net/clientaccesspolicy.xml"); //Create the cookie container and add a cookie. request.CookieContainer = new CookieContainer(); // This example shows manually adding a cookie, but you would most // likely read the cookies from isolated storage. request.CookieContainer.Add(new Uri("http://api.search.live.net"), new Cookie("id", "1234")); // Send the request. request.BeginGetResponse(new AsyncCallback(ReadCallback), request); } // Get the response and write cookies to isolated storage. private void ReadCallback(IAsyncResult asynchronousResult) { HttpWebRequest request = (HttpWebRequest)asynchronousResult.AsyncState; HttpWebResponse response = (HttpWebResponse) request.EndGetResponse(asynchronousResult); using (IsolatedStorageFile isf = IsolatedStorageFile.GetUserStoreForSite()) { using (IsolatedStorageFileStream isfs = isf.OpenFile("CookieExCookies", FileMode.OpenOrCreate, FileAccess.Write)) { using (StreamWriter sw = new StreamWriter(isfs)) { foreach (Cookie cookieValue in response.Cookies) { sw.WriteLine("Cookie: " + cookieValue.ToString()); } sw.Close(); } } } } private void ReadFromIsolatedStorage() { using (IsolatedStorageFile isf = IsolatedStorageFile.GetUserStoreForSite()) { using (IsolatedStorageFileStream isfs = isf.OpenFile("CookieExCookies", FileMode.Open)) { using (StreamReader sr = new StreamReader(isfs)) { tb1.Text = sr.ReadToEnd(); sr.Close(); } } } } } }
更多追问追答
追问
简述。
追答
不能再简了,再简的话,就不对的了。
推荐律师服务:
若未解决您的问题,请您详细描述您的问题,通过百度律临进行免费专业咨询