
android 中如何限制 EditText 最大输入字符数
2016-02-19 · 百度知道合伙人官方认证企业
1【专注:Python+人工智能|Java大数据|HTML5培训】 2【免费提供名师直播课堂、公开课及视频教程】 3【地址:北京市昌平区三旗百汇物美大卖场2层,微信公众号:yuzhitc】
向TA提问
关注
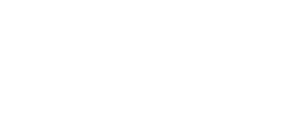
展开全部
方法一:
在 xml 文件中设置文本编辑框属性作字符数限制
如:android:maxLength="10" 即限制最大输入字符个数为10
方法二:
在代码中使用InputFilter 进行过滤
//editText.setFilters(new InputFilter[]{new InputFilter.LengthFilter(20)}); 即限定最大输入字符数为20
方法三:
利用 TextWatcher 进行监听
在 xml 文件中设置文本编辑框属性作字符数限制
如:android:maxLength="10" 即限制最大输入字符个数为10
方法二:
在代码中使用InputFilter 进行过滤
//editText.setFilters(new InputFilter[]{new InputFilter.LengthFilter(20)}); 即限定最大输入字符数为20
方法三:
利用 TextWatcher 进行监听
展开全部
方法一:
在 xml 文件中设置文本编辑框属性作字符数限制
如:android:maxLength="10" 即限制最大输入字符个数为10
方法二:
在代码中使用InputFilter 进行过滤
//editText.setFilters(new InputFilter[]{new InputFilter.LengthFilter(20)}); 即限定最大输入字符数为20
[java] view plaincopyprint?
01.public class TextEditActivity extends Activity {
02. /** Called when the activity is first created. */
03. @Override
04. public void onCreate(Bundle savedInstanceState) {
05. super.onCreate(savedInstanceState);
06. setContentView(R.layout.main);
07.
08. EditText editText = (EditText)findViewById(R.id.entry);
09. editText.setFilters(new InputFilter[]{new InputFilter.LengthFilter(20)});
10. }
11.}
public class TextEditActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
EditText editText = (EditText)findViewById(R.id.entry);
editText.setFilters(new InputFilter[]{new InputFilter.LengthFilter(20)});
}
}
方法三:
利用 TextWatcher 进行监听
[java] view plaincopyprint?
01.package cie.textEdit;
02.
03.import android.text.Editable;
04.import android.text.Selection;
05.import android.text.TextWatcher;
06.import android.widget.EditText;
07.
08./*
09. * 监听输入内容是否超出最大长度,并设置光标位置
10. * */
11.public class MaxLengthWatcher implements TextWatcher {
12.
13. private int maxLen = 0;
14. private EditText editText = null;
15.
16.
17. public MaxLengthWatcher(int maxLen, EditText editText) {
18. this.maxLen = maxLen;
19. this.editText = editText;
20. }
21.
22. public void afterTextChanged(Editable arg0) {
23. // TODO Auto-generated method stub
24.
25. }
26.
27. public void beforeTextChanged(CharSequence arg0, int arg1, int arg2,
28. int arg3) {
29. // TODO Auto-generated method stub
30.
31. }
32.
33. public void onTextChanged(CharSequence arg0, int arg1, int arg2, int arg3) {
34. // TODO Auto-generated method stub
35. Editable editable = editText.getText();
36. int len = editable.length();
37.
38. if(len > maxLen)
39. {
40. int selEndIndex = Selection.getSelectionEnd(editable);
41. String str = editable.toString();
42. //截取新字符串
43. String newStr = str.substring(0,maxLen);
44. editText.setText(newStr);
45. editable = editText.getText();
46.
47. //新字符串的长度
48. int newLen = editable.length();
49. //旧光标位置超过字符串长度
50. if(selEndIndex > newLen)
51. {
52. selEndIndex = editable.length();
53. }
54. //设置新光标所在的位置
55. Selection.setSelection(editable, selEndIndex);
56.
57. }
58. }
59.
60.}
package cie.textEdit;
import android.text.Editable;
import android.text.Selection;
import android.text.TextWatcher;
import android.widget.EditText;
/*
* 监听输入内容是否超出最大长度,并设置光标位置
* */
public class MaxLengthWatcher implements TextWatcher {
private int maxLen = 0;
private EditText editText = null;
public MaxLengthWatcher(int maxLen, EditText editText) {
this.maxLen = maxLen;
this.editText = editText;
}
public void afterTextChanged(Editable arg0) {
// TODO Auto-generated method stub
}
public void beforeTextChanged(CharSequence arg0, int arg1, int arg2,
int arg3) {
// TODO Auto-generated method stub
}
public void onTextChanged(CharSequence arg0, int arg1, int arg2, int arg3) {
// TODO Auto-generated method stub
Editable editable = editText.getText();
int len = editable.length();
if(len > maxLen)
{
int selEndIndex = Selection.getSelectionEnd(editable);
String str = editable.toString();
//截取新字符串
String newStr = str.substring(0,maxLen);
editText.setText(newStr);
editable = editText.getText();
//新字符串的长度
int newLen = editable.length();
//旧光标位置超过字符串长度
if(selEndIndex > newLen)
{
selEndIndex = editable.length();
}
//设置新光标所在的位置
Selection.setSelection(editable, selEndIndex);
}
}
}
对应的 activity 部分的调用为:
[java] view plaincopyprint?
01.package cie.textEdit;
02.
03.import android.app.Activity;
04.import android.os.Bundle;
05.import android.text.InputFilter;
06.import android.widget.EditText;
07.
08.public class TextEditActivity extends Activity {
09. /** Called when the activity is first created. */
10. @Override
11. public void onCreate(Bundle savedInstanceState) {
12. super.onCreate(savedInstanceState);
13. setContentView(R.layout.main);
14.
15. EditText editText = (EditText) findViewById(R.id.entry);
16. editText.addTextChangedListener(new MaxLengthWatcher(10, editText));
17.
18. }
19.}
package cie.textEdit;
import android.app.Activity;
import android.os.Bundle;
import android.text.InputFilter;
import android.widget.EditText;
public class TextEditActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
EditText editText = (EditText) findViewById(R.id.entry);
editText.addTextChangedListener(new MaxLengthWatcher(10, editText));
}
}
限制输入字符数为10个
main.xml 文件
[html] view plaincopyprint?
01.<?xml version="1.0" encoding="utf-8"?>
02.<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
03. android:layout_width="fill_parent"
04. android:layout_height="fill_parent">
05. <TextView
06. android:id="@+id/label"
07. android:layout_width="fill_parent"
08. android:layout_height="wrap_content"
09. android:text="Type here:"/>
10. <EditText
11. android:id="@+id/entry"
12. android:singleLine="true"
13. android:layout_width="fill_parent"
14. android:layout_height="wrap_content"
15. android:background="@android:drawable/editbox_background"
16. android:layout_below="@id/label"/>
17. <Button
18. android:id="@+id/ok"
19. android:layout_width="wrap_content"
20. android:layout_height="wrap_content"
21. android:layout_below="@id/entry"
22. android:layout_alignParentRight="true"
23. android:layout_marginLeft="10dip"
24. android:text="OK" />
25. <Button
26. android:layout_width="wrap_content"
27. android:layout_height="wrap_content"
28. android:layout_toLeftOf="@id/ok"
29. android:layout_alignTop="@id/ok"
30. android:text="Cancel" />
31.</RelativeLayout>
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TextView
android:id="@+id/label"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Type here:"/>
<EditText
android:id="@+id/entry"
android:singleLine="true"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:background="@android:drawable/editbox_background"
android:layout_below="@id/label"/>
<Button
android:id="@+id/ok"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@id/entry"
android:layout_alignParentRight="true"
android:layout_marginLeft="10dip"
android:text="OK" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_toLeftOf="@id/ok"
android:layout_alignTop="@id/ok"
android:text="Cancel" />
</RelativeLayout>
在 xml 文件中设置文本编辑框属性作字符数限制
如:android:maxLength="10" 即限制最大输入字符个数为10
方法二:
在代码中使用InputFilter 进行过滤
//editText.setFilters(new InputFilter[]{new InputFilter.LengthFilter(20)}); 即限定最大输入字符数为20
[java] view plaincopyprint?
01.public class TextEditActivity extends Activity {
02. /** Called when the activity is first created. */
03. @Override
04. public void onCreate(Bundle savedInstanceState) {
05. super.onCreate(savedInstanceState);
06. setContentView(R.layout.main);
07.
08. EditText editText = (EditText)findViewById(R.id.entry);
09. editText.setFilters(new InputFilter[]{new InputFilter.LengthFilter(20)});
10. }
11.}
public class TextEditActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
EditText editText = (EditText)findViewById(R.id.entry);
editText.setFilters(new InputFilter[]{new InputFilter.LengthFilter(20)});
}
}
方法三:
利用 TextWatcher 进行监听
[java] view plaincopyprint?
01.package cie.textEdit;
02.
03.import android.text.Editable;
04.import android.text.Selection;
05.import android.text.TextWatcher;
06.import android.widget.EditText;
07.
08./*
09. * 监听输入内容是否超出最大长度,并设置光标位置
10. * */
11.public class MaxLengthWatcher implements TextWatcher {
12.
13. private int maxLen = 0;
14. private EditText editText = null;
15.
16.
17. public MaxLengthWatcher(int maxLen, EditText editText) {
18. this.maxLen = maxLen;
19. this.editText = editText;
20. }
21.
22. public void afterTextChanged(Editable arg0) {
23. // TODO Auto-generated method stub
24.
25. }
26.
27. public void beforeTextChanged(CharSequence arg0, int arg1, int arg2,
28. int arg3) {
29. // TODO Auto-generated method stub
30.
31. }
32.
33. public void onTextChanged(CharSequence arg0, int arg1, int arg2, int arg3) {
34. // TODO Auto-generated method stub
35. Editable editable = editText.getText();
36. int len = editable.length();
37.
38. if(len > maxLen)
39. {
40. int selEndIndex = Selection.getSelectionEnd(editable);
41. String str = editable.toString();
42. //截取新字符串
43. String newStr = str.substring(0,maxLen);
44. editText.setText(newStr);
45. editable = editText.getText();
46.
47. //新字符串的长度
48. int newLen = editable.length();
49. //旧光标位置超过字符串长度
50. if(selEndIndex > newLen)
51. {
52. selEndIndex = editable.length();
53. }
54. //设置新光标所在的位置
55. Selection.setSelection(editable, selEndIndex);
56.
57. }
58. }
59.
60.}
package cie.textEdit;
import android.text.Editable;
import android.text.Selection;
import android.text.TextWatcher;
import android.widget.EditText;
/*
* 监听输入内容是否超出最大长度,并设置光标位置
* */
public class MaxLengthWatcher implements TextWatcher {
private int maxLen = 0;
private EditText editText = null;
public MaxLengthWatcher(int maxLen, EditText editText) {
this.maxLen = maxLen;
this.editText = editText;
}
public void afterTextChanged(Editable arg0) {
// TODO Auto-generated method stub
}
public void beforeTextChanged(CharSequence arg0, int arg1, int arg2,
int arg3) {
// TODO Auto-generated method stub
}
public void onTextChanged(CharSequence arg0, int arg1, int arg2, int arg3) {
// TODO Auto-generated method stub
Editable editable = editText.getText();
int len = editable.length();
if(len > maxLen)
{
int selEndIndex = Selection.getSelectionEnd(editable);
String str = editable.toString();
//截取新字符串
String newStr = str.substring(0,maxLen);
editText.setText(newStr);
editable = editText.getText();
//新字符串的长度
int newLen = editable.length();
//旧光标位置超过字符串长度
if(selEndIndex > newLen)
{
selEndIndex = editable.length();
}
//设置新光标所在的位置
Selection.setSelection(editable, selEndIndex);
}
}
}
对应的 activity 部分的调用为:
[java] view plaincopyprint?
01.package cie.textEdit;
02.
03.import android.app.Activity;
04.import android.os.Bundle;
05.import android.text.InputFilter;
06.import android.widget.EditText;
07.
08.public class TextEditActivity extends Activity {
09. /** Called when the activity is first created. */
10. @Override
11. public void onCreate(Bundle savedInstanceState) {
12. super.onCreate(savedInstanceState);
13. setContentView(R.layout.main);
14.
15. EditText editText = (EditText) findViewById(R.id.entry);
16. editText.addTextChangedListener(new MaxLengthWatcher(10, editText));
17.
18. }
19.}
package cie.textEdit;
import android.app.Activity;
import android.os.Bundle;
import android.text.InputFilter;
import android.widget.EditText;
public class TextEditActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
EditText editText = (EditText) findViewById(R.id.entry);
editText.addTextChangedListener(new MaxLengthWatcher(10, editText));
}
}
限制输入字符数为10个
main.xml 文件
[html] view plaincopyprint?
01.<?xml version="1.0" encoding="utf-8"?>
02.<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
03. android:layout_width="fill_parent"
04. android:layout_height="fill_parent">
05. <TextView
06. android:id="@+id/label"
07. android:layout_width="fill_parent"
08. android:layout_height="wrap_content"
09. android:text="Type here:"/>
10. <EditText
11. android:id="@+id/entry"
12. android:singleLine="true"
13. android:layout_width="fill_parent"
14. android:layout_height="wrap_content"
15. android:background="@android:drawable/editbox_background"
16. android:layout_below="@id/label"/>
17. <Button
18. android:id="@+id/ok"
19. android:layout_width="wrap_content"
20. android:layout_height="wrap_content"
21. android:layout_below="@id/entry"
22. android:layout_alignParentRight="true"
23. android:layout_marginLeft="10dip"
24. android:text="OK" />
25. <Button
26. android:layout_width="wrap_content"
27. android:layout_height="wrap_content"
28. android:layout_toLeftOf="@id/ok"
29. android:layout_alignTop="@id/ok"
30. android:text="Cancel" />
31.</RelativeLayout>
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TextView
android:id="@+id/label"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Type here:"/>
<EditText
android:id="@+id/entry"
android:singleLine="true"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:background="@android:drawable/editbox_background"
android:layout_below="@id/label"/>
<Button
android:id="@+id/ok"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@id/entry"
android:layout_alignParentRight="true"
android:layout_marginLeft="10dip"
android:text="OK" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_toLeftOf="@id/ok"
android:layout_alignTop="@id/ok"
android:text="Cancel" />
</RelativeLayout>
本回答被提问者采纳
已赞过
已踩过<
评论
收起
你对这个回答的评价是?
推荐律师服务:
若未解决您的问题,请您详细描述您的问题,通过百度律临进行免费专业咨询