
android拍照+文字上传到.net或webservice怎么做
2015-02-10 · 知道合伙人数码行家
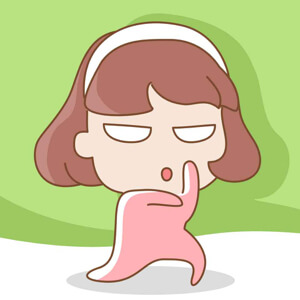
知道合伙人数码行家
向TA提问 私信TA
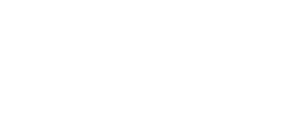
1
在eclipse上安装好android开发环境,android调用webservice使用ksoap,本经验使用的是ksoap2-android-assembly-2.6.4-jar-with-dependencies.jar
2
AndroidManifest.xml中需添加相应的权限,例子:
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.camera"
android:versionCode="1"
android:versionName="1.0" >
<uses-sdk
android:minSdkVersion="8"
android:targetSdkVersion="9" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/>
<uses-permission android:name="android.permission.MOUNT_UNMOUNT_FILESYSTEMS"/>
<uses-permission android:name="android.permission.INTERNET"/>
<application
android:allowBackup="true"
android:icon="@drawable/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme" >
<activity
android:name="com.example.camera.MainActivity"
android:label="@string/app_name" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
3
activity_main.xml文件代码
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical">
<Button
android:id="@+id/button"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="点击启动相机"/>
<Button
android:id="@+id/savePic"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="保存图片到服务器"/>
<TextView
android:id="@+id/textView"
android:layout_width="fill_parent"
android:layout_height="wrap_content"/>
<ImageView
android:id="@+id/imageView"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:background="#999999" />
</LinearLayout>
4
MainActivity具体代码
package com.example.camera;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import org.kobjects.base64.Base64;
import org.ksoap2.SoapEnvelope;
import org.ksoap2.serialization.SoapObject;
import org.ksoap2.serialization.SoapSerializationEnvelope;
import org.ksoap2.transport.HttpTransportSE;
import android.app.Activity;
import android.content.Intent;
import android.graphics.Bitmap;
import android.os.Bundle;
import android.os.Environment;
import android.provider.MediaStore;
import android.util.Log;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.ImageView;
import android.widget.TextView;
public class MainActivity extends Activity {
TextView tv = null;
String fileName = "/sdcard/myImage/my.jpg"; //图片保存sd地址
String namespace = "http://webservice.service.com"; // 命名空间
String url = "http://192.168.200.19:8080/Web/webservices/Portal"; //对应的url
String methodName = "uploadImage"; //webservice方法
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
tv = (TextView)findViewById(R.id.textView);
//启用相机按钮
Button button = (Button) findViewById(R.id.button);
button.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
Intent intent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
startActivityForResult(intent, 1);
}
});
//保存图片到服务器按钮(通过webservice实现)
Button saveButton = (Button) findViewById(R.id.savePic);
saveButton.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
testUpload();
}
});
}
/**
* 拍照完成后,回掉的方法
*/
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (resultCode == Activity.RESULT_OK) {
String sdStatus = Environment.getExternalStorageState();
if (!sdStatus.equals(Environment.MEDIA_MOUNTED)) { // 检测sd是否可用
Log.v("TestFile",
"SD card is not avaiable/writeable right now.");
return;
}
Bundle bundle = data.getExtras();
Bitmap bitmap = (Bitmap) bundle.get("data");// 获取相机返回的数据,并转换为Bitmap图片格式
FileOutputStream b = null;
File file = new File("/sdcard/myImage/");
file.mkdirs();// 创建文件夹
try {
b = new FileOutputStream(fileName);
bitmap.compress(Bitmap.CompressFormat.JPEG, 100, b);// 把数据写入文件
} catch (FileNotFoundException e) {
e.printStackTrace();
} finally {
try {
b.flush();
b.close();
} catch (IOException e) {
e.printStackTrace();
}
}
((ImageView) findViewById(R.id.imageView)).setImageBitmap(bitmap);// 将图片显示在ImageView里
}
}
/**
* 图片上传方法
*
* 1.把图片信息通过Base64转换成字符串
* 2.调用connectWebService方法实现上传
*/
private void testUpload(){
try{
FileInputStream fis = new FileInputStream(fileName);
ByteArrayOutputStream baos = new ByteArrayOutputStream();
byte[] buffer = new byte[1024];
int count = 0;
while((count = fis.read(buffer)) >= 0){
baos.write(buffer, 0, count);
}
String uploadBuffer = new String(Base64.encode(baos.toByteArray())); //进行Base64编码
connectWebService(uploadBuffer);
Log.i("connectWebService", "start");
fis.close();
}catch(Exception e){
e.printStackTrace();
}
}
/**
* 通过webservice实现图片上传
*
* @param imageBuffer
*/
private void connectWebService(String imageBuffer) {
//以下就是 调用过程了,不明白的话 请看相关webservice文档
SoapObject soapObject = new SoapObject(namespace, methodName);
soapObject.addProperty("filename", "my.jpg"); //参数1 图片名
soapObject.addProperty("image", imageBuffer); //参数2 图片字符串
SoapSerializationEnvelope envelope = new SoapSerializationEnvelope(
SoapEnvelope.VER11);
envelope.dotNet = false;
envelope.setOutputSoapObject(soapObject);
HttpTransportSE httpTranstation = new HttpTransportSE(url);
try {
httpTranstation.call(namespace, envelope);
Object result = envelope.getResponse();
Log.i("connectWebService", result.toString());
tv.setText(result.toString());
} catch (Exception e) {
e.printStackTrace();
tv.setText(e.getStackTrace().toString());
}
}
}
5
服务端webservice方法(cxf)
public String uploadImage(String filename, String image) {
FileOutputStream fos = null;
try{
String toDir = "D:\\work\\image"; //存储路径
byte[] buffer = new BASE64Decoder().decodeBuffer(image); //对android传过来的图片字符串进行解码
File destDir = new File(toDir);
if(!destDir.exists()) {
destDir.mkdir();
}
fos = new FileOutputStream(new File(destDir,filename)); //保存图片
fos.write(buffer);
fos.flush();
fos.close();
return "上传图片成功!" + "图片路径为:" + toDir;
}catch (Exception e){
e.printStackTrace();
}
return "上传图片失败!";
}
操作步骤
(1)点击“点击启动相机”按钮,回到拍照模式下,拍照完成,回到初始界面,显示刚拍的照片
(2)点击“保存照片到服务器”按钮,通过webservice把照片保存到服务器中,TextView显示保存信息。