
C++中const变量难道能随便赋值吗?
如题。下面这个程序来自《面向对象程序设计(C++语言描述)》第2.7节例题。我的问题是(1)函数print_elephants和free_list的参数为什么一定要写成c...
如题。下面这个程序来自《面向对象程序设计(C++语言描述)》第2.7节例题。我的问题是(1)函数print_elephants和free_list的参数为什么一定要写成const Elephant* ptr而不能只写Elephant* ptr呢?(2)比如free_list的函数体中,temp_ptr和ptr的类型都是const Elephant* ptr,可是却有这样的语句temp_ptr=ptr->next,难道说const类型的变量可以随便赋值么?
#include <iostream>
#include <string>
using namespace std;
struct Elephant{
string name;
Elephant* next;
};
void print_elephants(const Elephant* ptr);
Elephant* get_elephants();
void free_list(const Elephant* ptr);
int main(){
Elephant* start;
start=get_elephants();
print_elephants(start);
free_list(start);
return 0;
}
//get_elephants dynamically allocates storage
//for nodes.It builds the linked list and
//stores user-supplied names in the name
//member of the nodes.It returns a pointer
//to the first such node.
Elephant* get_elephants(){
Elephant *current,*first;
int response;
//allocate first node
current=first=new Elephant;
//store name for first Elephant
cout<<"\nNAME: ";
cin>>current->name;
//prompt user about another Elephant
cout<<"\nAdd another?(1==yes,0==no):";
cin>>response;
//Add Elephants to list until user signals halt.
while(response==1){
//allocate another Elephant node
current=current->next=new Elephant;
//store name of next Elephant
cout<<"\nNAME: ";
cin>>current->name;
//prompt user about another Elephant
cout<<"\nAdd another?(1=yew,0==no): ";
cin>>response;
}
//set link field in last node to 0
current->next=0;
return first;
}
//print_elephants steps through the linked
//list pointer to by ptr and prints the name
//of the node in the list
void print_elephants(const Elephant* ptr){
int count=1;
cout<<"\n\n\n";
while(ptr!=0){
cout<<"Elephant number "<<count++
<<"is "<<ptr->name<<'\n';
ptr=ptr->next;
}
}
//free_list steps through the linked list pointed
//to by ptr and frees each node in the list
void free_list(const Elephant* ptr){
const Elephant* temp_ptr;
while(ptr!=0){
temp_ptr=ptr->next;
delete ptr;
ptr=temp_ptr;
}
}
请达人指点,谢谢了! 展开
#include <iostream>
#include <string>
using namespace std;
struct Elephant{
string name;
Elephant* next;
};
void print_elephants(const Elephant* ptr);
Elephant* get_elephants();
void free_list(const Elephant* ptr);
int main(){
Elephant* start;
start=get_elephants();
print_elephants(start);
free_list(start);
return 0;
}
//get_elephants dynamically allocates storage
//for nodes.It builds the linked list and
//stores user-supplied names in the name
//member of the nodes.It returns a pointer
//to the first such node.
Elephant* get_elephants(){
Elephant *current,*first;
int response;
//allocate first node
current=first=new Elephant;
//store name for first Elephant
cout<<"\nNAME: ";
cin>>current->name;
//prompt user about another Elephant
cout<<"\nAdd another?(1==yes,0==no):";
cin>>response;
//Add Elephants to list until user signals halt.
while(response==1){
//allocate another Elephant node
current=current->next=new Elephant;
//store name of next Elephant
cout<<"\nNAME: ";
cin>>current->name;
//prompt user about another Elephant
cout<<"\nAdd another?(1=yew,0==no): ";
cin>>response;
}
//set link field in last node to 0
current->next=0;
return first;
}
//print_elephants steps through the linked
//list pointer to by ptr and prints the name
//of the node in the list
void print_elephants(const Elephant* ptr){
int count=1;
cout<<"\n\n\n";
while(ptr!=0){
cout<<"Elephant number "<<count++
<<"is "<<ptr->name<<'\n';
ptr=ptr->next;
}
}
//free_list steps through the linked list pointed
//to by ptr and frees each node in the list
void free_list(const Elephant* ptr){
const Elephant* temp_ptr;
while(ptr!=0){
temp_ptr=ptr->next;
delete ptr;
ptr=temp_ptr;
}
}
请达人指点,谢谢了! 展开
展开全部
1)函数print_elephants和free_list的参数为什么一定要写成const Elephant* ptr而不能只写Elephant* ptr呢?
答:保证传入内容不被修改。
(2)比如free_list的函数体中,temp_ptr和ptr的类型都是const Elephant* ptr,可是却有这样的语句temp_ptr=ptr->next,难道说const类型的变量可以随便赋值么
答:定义的是常量指针,不是常量值,常量指针指向的内容是不可以被修改的。
不知还有什么疑问~~~
就这么回事。
#include <iostream>
using namespace std;
int main()
{
const int *p;
int *q;
int a,b;
scanf("%d %d", &a,&b);
p = &a;
q = &b;
*q = 3;
*p = 2;
printf("a = %d,b = %d \n",a,b);
return 0;
}
*p 修改不了a的值就是因为p是常指针。
2015-12-05 · 做真实的自己 用良心做教育
千锋教育专注HTML5大前端、JavaEE、Python、人工智能、UI&UE、云计算、全栈软件测试、大数据、物联网+嵌入式、Unity游戏开发、网络安全、互联网营销、Go语言等培训教育。
向TA提问
关注
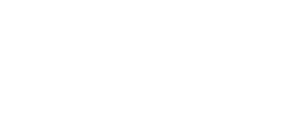
展开全部
不是的,在对象生存期内是不可以改变的,过了生存期就是普通变量了。
在C++中,const成员变量也不能在类定义处初始化,只能通过构造函数初始化列表进行,并且必须有构造函数。
const数据成员只在某个对象生存期内是常量,而对于整个类而言却是可变的。因为类可以创建多个对象,不同的对象其const数据成员的值可以不同。所以不能在类的声明中初始化const数据成员,因为类的对象没被创建时,编译器不知道const数据成员的值是什么。
const数据成员的初始化只能在类的构造函数的初始化列表中进行。要想建立在整个类中都恒定的常量,应该用类中的枚举常量来实现,或者static cosnt。
class Test
{
public:
Test():a(0){}
enum {size1=100,size2=200};
private:
const int a;//只能在构造函数初始化列表中初始化
static int b;//在类的实现文件中定义并初始化
const static int c;//与 static const int c;相同。
};
int Test::b=0;//static成员变量不能在构造函数初始化列表中初始化,因为它不属于某个对象。
cosnt int Test::c=0;//注意:给静态成员变量赋值时,不需要加static修饰符。但要加cosnt
在C++中,const成员变量也不能在类定义处初始化,只能通过构造函数初始化列表进行,并且必须有构造函数。
const数据成员只在某个对象生存期内是常量,而对于整个类而言却是可变的。因为类可以创建多个对象,不同的对象其const数据成员的值可以不同。所以不能在类的声明中初始化const数据成员,因为类的对象没被创建时,编译器不知道const数据成员的值是什么。
const数据成员的初始化只能在类的构造函数的初始化列表中进行。要想建立在整个类中都恒定的常量,应该用类中的枚举常量来实现,或者static cosnt。
class Test
{
public:
Test():a(0){}
enum {size1=100,size2=200};
private:
const int a;//只能在构造函数初始化列表中初始化
static int b;//在类的实现文件中定义并初始化
const static int c;//与 static const int c;相同。
};
int Test::b=0;//static成员变量不能在构造函数初始化列表中初始化,因为它不属于某个对象。
cosnt int Test::c=0;//注意:给静态成员变量赋值时,不需要加static修饰符。但要加cosnt
本回答被网友采纳
已赞过
已踩过<
评论
收起
你对这个回答的评价是?
2010-02-10
展开全部
const Elephant* ptr
这样是为了防止修改ptr指针指向的地址保存的值,如果你可以确保在这个函数中没有出现意外的修改ptr指向的内容,那么也可以不加const
而且这里的const不是对ptr而言的,而是对ptr指向的内容而言的,所以ptr还可以指向其它地址,如果是以下形式,那么ptr就不能指向其它地址了:
Elephant * const ptr
这种形式的定义说明,ptr是一个只读指针,它的指向不能更改,但是指向的内容可以更改,如果指向的地址和指向的地址保存的值都不想更改,就用以下形式:
const Elephant * const ptr
这样是为了防止修改ptr指针指向的地址保存的值,如果你可以确保在这个函数中没有出现意外的修改ptr指向的内容,那么也可以不加const
而且这里的const不是对ptr而言的,而是对ptr指向的内容而言的,所以ptr还可以指向其它地址,如果是以下形式,那么ptr就不能指向其它地址了:
Elephant * const ptr
这种形式的定义说明,ptr是一个只读指针,它的指向不能更改,但是指向的内容可以更改,如果指向的地址和指向的地址保存的值都不想更改,就用以下形式:
const Elephant * const ptr
已赞过
已踩过<
评论
收起
你对这个回答的评价是?
展开全部
(1) 这两个函数用 const Elephant* ptr 做参数一是让读者明白这两个函数不会改变指针所指向的内容;二是防止编程的误操作修改ptr指针指向的内容。
(2) const Elephant* temp_ptr 表示temp_ptr 指向的内容是常量不可改变,但是temp_ptr 本身可变,也就是可以temp_ptr = ptr->next 是正确的,但是*temp_ptr = 15 这样的赋值操作就是错误的。
PS:
Elephant * const temp_ptr 这样的定义是指针不可变,自始至终只能指向一块内存空间
const Elephant * const temp_ptr 这样定义是指针和指针指向的内容都不能改变。
(2) const Elephant* temp_ptr 表示temp_ptr 指向的内容是常量不可改变,但是temp_ptr 本身可变,也就是可以temp_ptr = ptr->next 是正确的,但是*temp_ptr = 15 这样的赋值操作就是错误的。
PS:
Elephant * const temp_ptr 这样的定义是指针不可变,自始至终只能指向一块内存空间
const Elephant * const temp_ptr 这样定义是指针和指针指向的内容都不能改变。
已赞过
已踩过<
评论
收起
你对这个回答的评价是?
展开全部
这么长的程序,你有疑问在哪一行代码也不指出,真想让人从头到尾的看你的程序啊???
已赞过
已踩过<
评论
收起
你对这个回答的评价是?
推荐律师服务:
若未解决您的问题,请您详细描述您的问题,通过百度律临进行免费专业咨询