
Android手机怎么获取power
2016-02-04 · 百度知道合伙人官方认证企业
1【专注:Python+人工智能|Java大数据|HTML5培训】 2【免费提供名师直播课堂、公开课及视频教程】 3【地址:北京市昌平区三旗百汇物美大卖场2层,微信公众号:yuzhitc】
向TA提问
关注
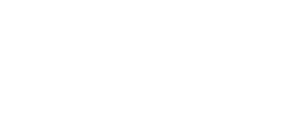
展开全部
这个是获取电量的android DEMO:
package com.android.batterywaster;
import android.app.Activity;
import android.content.BroadcastReceiver;
import android.content.Context;
import android.content.Intent;
import android.content.IntentFilter;
import android.os.BatteryManager;
import android.os.Bundle;
import android.os.PowerManager;
import android.view.View;
import android.widget.CheckBox;
import android.widget.TextView;
import java.text.DateFormat;
import java.util.Date;
/**
* So you thought sync used up your battery life.
*/
public class BatteryWaster extends Activity {
TextView mLog;
DateFormat mDateFormat;
IntentFilter mFilter;
PowerManager.WakeLock mWakeLock;
SpinThread mThread;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// Set the layout for this activity. You can find it
// in res/layout/hello_activity.xml
setContentView(R.layout.main);
findViewById(R.id.checkbox).setOnClickListener(mClickListener);
mLog = (TextView)findViewById(R.id.log);
mDateFormat = DateFormat.getInstance();
mFilter = new IntentFilter();
mFilter.addAction(Intent.ACTION_BATTERY_CHANGED);
mFilter.addAction(Intent.ACTION_BATTERY_LOW);
mFilter.addAction(Intent.ACTION_BATTERY_OKAY);
mFilter.addAction(Intent.ACTION_POWER_CONNECTED);
PowerManager pm = (PowerManager)getSystemService(POWER_SERVICE);
mWakeLock = pm.newWakeLock(PowerManager.FULL_WAKE_LOCK, "BatteryWaster");
mWakeLock.setReferenceCounted(false);
}
@Override
public void onPause() {
stopRunning();
}
View.OnClickListener mClickListener = new View.OnClickListener() {
public void onClick(View v) {
CheckBox checkbox = (CheckBox)v;
if (checkbox.isChecked()) {
startRunning();
} else {
stopRunning();
}
}
};
void startRunning() {
log("Start");
registerReceiver(mReceiver, mFilter);
mWakeLock.acquire();
if (mThread == null) {
mThread = new SpinThread();
mThread.start();
}
}
void stopRunning() {
log("Stop");
unregisterReceiver(mReceiver);
mWakeLock.release();
if (mThread != null) {
mThread.quit();
mThread = null;
}
}
void log(String s) {
mLog.setText(mLog.getText() + "\n" + mDateFormat.format(new Date()) + ": " + s);
}
BroadcastReceiver mReceiver = new BroadcastReceiver() {
public void onReceive(Context context, Intent intent) {
String action = intent.getAction();
String title = action;
int index = title.lastIndexOf('.');
if (index >= 0) {
title = title.substring(index + 1);
}
if (Intent.ACTION_BATTERY_CHANGED.equals(action)) {
int level = intent.getIntExtra(BatteryManager.EXTRA_LEVEL, -1);
int icon = intent.getIntExtra(BatteryManager.EXTRA_ICON_SMALL,-1);
log(title + ": level=" + level + "\n" + "icon:" + icon);
} else {
log(title);
}
}
};
class SpinThread extends Thread {
private boolean mStop;
public void quit() {
synchronized (this) {
mStop = true;
}
}
public void run() {
while (true) {
synchronized (this) {
if (mStop) {
return;
}
}
}
}
}
}
这个是layout:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical"
>
<CheckBox android:id="@+id/checkbox"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_marginLeft="25dp"
android:layout_marginTop="25dp"
android:textSize="18sp"
android:textColor="#ffffffff"
android:text="@string/waste_away"
/>
<ScrollView android:id="@+id/scroll"
android:layout_width="fill_parent"
android:layout_height="0px"
android:layout_weight="1"
>
<TextView android:id="@+id/log"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_marginTop="25dp"
android:textSize="12sp"
android:textColor="#ffffffff"
/>
</ScrollView>
</LinearLayout>
package com.android.batterywaster;
import android.app.Activity;
import android.content.BroadcastReceiver;
import android.content.Context;
import android.content.Intent;
import android.content.IntentFilter;
import android.os.BatteryManager;
import android.os.Bundle;
import android.os.PowerManager;
import android.view.View;
import android.widget.CheckBox;
import android.widget.TextView;
import java.text.DateFormat;
import java.util.Date;
/**
* So you thought sync used up your battery life.
*/
public class BatteryWaster extends Activity {
TextView mLog;
DateFormat mDateFormat;
IntentFilter mFilter;
PowerManager.WakeLock mWakeLock;
SpinThread mThread;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// Set the layout for this activity. You can find it
// in res/layout/hello_activity.xml
setContentView(R.layout.main);
findViewById(R.id.checkbox).setOnClickListener(mClickListener);
mLog = (TextView)findViewById(R.id.log);
mDateFormat = DateFormat.getInstance();
mFilter = new IntentFilter();
mFilter.addAction(Intent.ACTION_BATTERY_CHANGED);
mFilter.addAction(Intent.ACTION_BATTERY_LOW);
mFilter.addAction(Intent.ACTION_BATTERY_OKAY);
mFilter.addAction(Intent.ACTION_POWER_CONNECTED);
PowerManager pm = (PowerManager)getSystemService(POWER_SERVICE);
mWakeLock = pm.newWakeLock(PowerManager.FULL_WAKE_LOCK, "BatteryWaster");
mWakeLock.setReferenceCounted(false);
}
@Override
public void onPause() {
stopRunning();
}
View.OnClickListener mClickListener = new View.OnClickListener() {
public void onClick(View v) {
CheckBox checkbox = (CheckBox)v;
if (checkbox.isChecked()) {
startRunning();
} else {
stopRunning();
}
}
};
void startRunning() {
log("Start");
registerReceiver(mReceiver, mFilter);
mWakeLock.acquire();
if (mThread == null) {
mThread = new SpinThread();
mThread.start();
}
}
void stopRunning() {
log("Stop");
unregisterReceiver(mReceiver);
mWakeLock.release();
if (mThread != null) {
mThread.quit();
mThread = null;
}
}
void log(String s) {
mLog.setText(mLog.getText() + "\n" + mDateFormat.format(new Date()) + ": " + s);
}
BroadcastReceiver mReceiver = new BroadcastReceiver() {
public void onReceive(Context context, Intent intent) {
String action = intent.getAction();
String title = action;
int index = title.lastIndexOf('.');
if (index >= 0) {
title = title.substring(index + 1);
}
if (Intent.ACTION_BATTERY_CHANGED.equals(action)) {
int level = intent.getIntExtra(BatteryManager.EXTRA_LEVEL, -1);
int icon = intent.getIntExtra(BatteryManager.EXTRA_ICON_SMALL,-1);
log(title + ": level=" + level + "\n" + "icon:" + icon);
} else {
log(title);
}
}
};
class SpinThread extends Thread {
private boolean mStop;
public void quit() {
synchronized (this) {
mStop = true;
}
}
public void run() {
while (true) {
synchronized (this) {
if (mStop) {
return;
}
}
}
}
}
}
这个是layout:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical"
>
<CheckBox android:id="@+id/checkbox"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_marginLeft="25dp"
android:layout_marginTop="25dp"
android:textSize="18sp"
android:textColor="#ffffffff"
android:text="@string/waste_away"
/>
<ScrollView android:id="@+id/scroll"
android:layout_width="fill_parent"
android:layout_height="0px"
android:layout_weight="1"
>
<TextView android:id="@+id/log"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_marginTop="25dp"
android:textSize="12sp"
android:textColor="#ffffffff"
/>
</ScrollView>
</LinearLayout>
2016-02-01
展开全部
这个是获取电量的android DEMO:
package com.android.batterywaster;
import android.app.Activity;
import android.content.BroadcastReceiver;
import android.content.Context;
import android.content.Intent;
import android.content.IntentFilter;
import android.os.BatteryManager;
import android.os.Bundle;
import android.os.PowerManager;
import android.view.View;
import android.widget.CheckBox;
import android.widget.TextView;
import java.text.DateFormat;
import java.util.Date;
/**
* So you thought sync used up your battery life.
*/
public class BatteryWaster extends Activity {
TextView mLog;
DateFormat mDateFormat;
IntentFilter mFilter;
PowerManager.WakeLock mWakeLock;
SpinThread mThread;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// Set the layout for this activity. You can find it
// in res/layout/hello_activity.xml
setContentView(R.layout.main);
findViewById(R.id.checkbox).setOnClickListener(mClickListener);
mLog = (TextView)findViewById(R.id.log);
mDateFormat = DateFormat.getInstance();
mFilter = new IntentFilter();
mFilter.addAction(Intent.ACTION_BATTERY_CHANGED);
mFilter.addAction(Intent.ACTION_BATTERY_LOW);
mFilter.addAction(Intent.ACTION_BATTERY_OKAY);
mFilter.addAction(Intent.ACTION_POWER_CONNECTED);
PowerManager pm = (PowerManager)getSystemService(POWER_SERVICE);
mWakeLock = pm.newWakeLock(PowerManager.FULL_WAKE_LOCK, "BatteryWaster");
mWakeLock.setReferenceCounted(false);
}
@Override
public void onPause() {
stopRunning();
}
View.OnClickListener mClickListener = new View.OnClickListener() {
public void onClick(View v) {
CheckBox checkbox = (CheckBox)v;
if (checkbox.isChecked()) {
startRunning();
} else {
stopRunning();
}
}
};
void startRunning() {
log("Start");
registerReceiver(mReceiver, mFilter);
mWakeLock.acquire();
if (mThread == null) {
mThread = new SpinThread();
mThread.start();
}
}
void stopRunning() {
log("Stop");
unregisterReceiver(mReceiver);
mWakeLock.release();
if (mThread != null) {
mThread.quit();
mThread = null;
}
}
void log(String s) {
mLog.setText(mLog.getText() + "\n" + mDateFormat.format(new Date()) + ": " + s);
}
BroadcastReceiver mReceiver = new BroadcastReceiver() {
public void onReceive(Context context, Intent intent) {
String action = intent.getAction();
String title = action;
int index = title.lastIndexOf('.');
if (index >= 0) {
title = title.substring(index + 1);
}
if (Intent.ACTION_BATTERY_CHANGED.equals(action)) {
int level = intent.getIntExtra(BatteryManager.EXTRA_LEVEL, -1);
int icon = intent.getIntExtra(BatteryManager.EXTRA_ICON_SMALL,-1);
log(title + ": level=" + level + "\n" + "icon:" + icon);
} else {
log(title);
}
}
};
class SpinThread extends Thread {
private boolean mStop;
public void quit() {
synchronized (this) {
mStop = true;
}
}
public void run() {
while (true) {
synchronized (this) {
if (mStop) {
return;
}
}
}
}
}
}
这个是layout:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical"
>
<CheckBox android:id="@+id/checkbox"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_marginLeft="25dp"
android:layout_marginTop="25dp"
android:textSize="18sp"
android:textColor="#ffffffff"
android:text="@string/waste_away"
/>
<ScrollView android:id="@+id/scroll"
android:layout_width="fill_parent"
android:layout_height="0px"
android:layout_weight="1"
>
<TextView android:id="@+id/log"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_marginTop="25dp"
android:textSize="12sp"
android:textColor="#ffffffff"
/>
</ScrollView>
</LinearLayout>
package com.android.batterywaster;
import android.app.Activity;
import android.content.BroadcastReceiver;
import android.content.Context;
import android.content.Intent;
import android.content.IntentFilter;
import android.os.BatteryManager;
import android.os.Bundle;
import android.os.PowerManager;
import android.view.View;
import android.widget.CheckBox;
import android.widget.TextView;
import java.text.DateFormat;
import java.util.Date;
/**
* So you thought sync used up your battery life.
*/
public class BatteryWaster extends Activity {
TextView mLog;
DateFormat mDateFormat;
IntentFilter mFilter;
PowerManager.WakeLock mWakeLock;
SpinThread mThread;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// Set the layout for this activity. You can find it
// in res/layout/hello_activity.xml
setContentView(R.layout.main);
findViewById(R.id.checkbox).setOnClickListener(mClickListener);
mLog = (TextView)findViewById(R.id.log);
mDateFormat = DateFormat.getInstance();
mFilter = new IntentFilter();
mFilter.addAction(Intent.ACTION_BATTERY_CHANGED);
mFilter.addAction(Intent.ACTION_BATTERY_LOW);
mFilter.addAction(Intent.ACTION_BATTERY_OKAY);
mFilter.addAction(Intent.ACTION_POWER_CONNECTED);
PowerManager pm = (PowerManager)getSystemService(POWER_SERVICE);
mWakeLock = pm.newWakeLock(PowerManager.FULL_WAKE_LOCK, "BatteryWaster");
mWakeLock.setReferenceCounted(false);
}
@Override
public void onPause() {
stopRunning();
}
View.OnClickListener mClickListener = new View.OnClickListener() {
public void onClick(View v) {
CheckBox checkbox = (CheckBox)v;
if (checkbox.isChecked()) {
startRunning();
} else {
stopRunning();
}
}
};
void startRunning() {
log("Start");
registerReceiver(mReceiver, mFilter);
mWakeLock.acquire();
if (mThread == null) {
mThread = new SpinThread();
mThread.start();
}
}
void stopRunning() {
log("Stop");
unregisterReceiver(mReceiver);
mWakeLock.release();
if (mThread != null) {
mThread.quit();
mThread = null;
}
}
void log(String s) {
mLog.setText(mLog.getText() + "\n" + mDateFormat.format(new Date()) + ": " + s);
}
BroadcastReceiver mReceiver = new BroadcastReceiver() {
public void onReceive(Context context, Intent intent) {
String action = intent.getAction();
String title = action;
int index = title.lastIndexOf('.');
if (index >= 0) {
title = title.substring(index + 1);
}
if (Intent.ACTION_BATTERY_CHANGED.equals(action)) {
int level = intent.getIntExtra(BatteryManager.EXTRA_LEVEL, -1);
int icon = intent.getIntExtra(BatteryManager.EXTRA_ICON_SMALL,-1);
log(title + ": level=" + level + "\n" + "icon:" + icon);
} else {
log(title);
}
}
};
class SpinThread extends Thread {
private boolean mStop;
public void quit() {
synchronized (this) {
mStop = true;
}
}
public void run() {
while (true) {
synchronized (this) {
if (mStop) {
return;
}
}
}
}
}
}
这个是layout:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical"
>
<CheckBox android:id="@+id/checkbox"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_marginLeft="25dp"
android:layout_marginTop="25dp"
android:textSize="18sp"
android:textColor="#ffffffff"
android:text="@string/waste_away"
/>
<ScrollView android:id="@+id/scroll"
android:layout_width="fill_parent"
android:layout_height="0px"
android:layout_weight="1"
>
<TextView android:id="@+id/log"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_marginTop="25dp"
android:textSize="12sp"
android:textColor="#ffffffff"
/>
</ScrollView>
</LinearLayout>
本回答被网友采纳
已赞过
已踩过<
评论
收起
你对这个回答的评价是?
展开全部
百度搜索一键获取root权限
已赞过
已踩过<
评论
收起
你对这个回答的评价是?
推荐律师服务:
若未解决您的问题,请您详细描述您的问题,通过百度律临进行免费专业咨询