
如何使用jQuery Draggable和Droppable实现拖拽功能
1个回答
2015-06-01 · 百度知道合伙人官方认证企业
关注
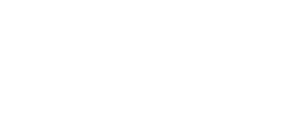
展开全部
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>jQuery UI Draggable and Droppable Demo</title>
<style type="text/css">
/*reset style*/
body {
color: #333;
font-size: 14px;
line-height: 22px;
font-family: "Helvetica","Arial","微软雅黑","宋体";
}
ul, li, p {
padding: 0;
margin: 0;
}
a {
color: #0064a0;
padding: 1px;
text-decoration: none;
}
a:hover {
color: #26a0da;
text-decoration: underline;
}
#leftpanel {
float: left;
}
.peopletabs li {
list-style-type: none;
}
#maincontainer {
width: 800px;
height: 350px;
margin: 0 auto;
border: 2px solid #0069a0;
padding: 10px;
}
#schoolproperty {
height: 250px;
border: 1px solid green;
padding: 10px;
overflow: auto;
width: 350px;
float: left;
}
#selectedschool {
float: right;
}
#rightlistcontainer {
width: 300px;
height: 250px;
overflow: auto;
float: right;
border: 1px solid green;
padding: 10px;
}
#peopleproperty ul {
list-style-type: none;
}
.ptreelist li {
display: block;
width: 200px;
}
/*拖动的元素最好设置一个宽带和高度*/
#draggableDiv {
z-index: 1000;
background-color: Green;
width: 308px;
height: 22px;
position: absolute;
color: #fff;
}
#middlepanel {
width: 100px;
float: left;
margin-top: 120px;
}
#rightlistcontainer .remove {
padding: 5px;
border: 1px solid #b4b4b4;
background: #cdcdcd;
}
#rightlistcontainer .remove li {
width: 240px;
background-color: #cdcdcd;
padding: 0 5px;
background: #fff;
margin-top: 3px;
cursor: move;
}
#blankarea {
margin-top:10px;
height: 50px;
border: 1px dotted red;
}
</style>
<script src="http://code.jquery.com/jquery-1.9.1.js"></script>
<script src="http://code.jquery.com/ui/1.10.3/jquery-ui.js"></script>
</head>
<body>
<div id="maincontainer">
<div id='draggableDiv' class="ui-widget-content">
</div>
<span class="closeBar" style="float: right"><a href="return false;" title="关闭">X</a></span>
<div id="contentcontainer">
<!--左边面板-->
<div id="leftpanel">
<ul class="peopletabs">
<li><a class="current" href="#" panel-id="schoolproperty">高校</a></li>
<!--<li><a href="#" panel-id="behavior_list">Tab2</a></li>
<li><a href="#" panel-id="productintent_list">Tab3</a></li>-->
</ul>
<div id="schoolproperty" class="threepanels">
<ul class="ptreelist">
<li id="44">江西高校<span class="closebutton"></span>
<ul class="ptreelist">
<li id="4407">江西科技师范学院<span class="closebutton"></span></li>
</ul>
<ul class="ptreelist">
<li id="4408">江西科技学院<span class="closebutton"></span></li>
</ul>
</li>
</ul>
</div>
</div>
<div id="middlepanel">
<p>可以从左侧拖动条件到右侧</p>
</div>
<!--右边面板-->
<div id="selectedschool">
<p>
符合条件的学生数<span id="peopleaccount" class="red" style="font-size: 20px">1000</span>人
</p>
<div id="rightlistcontainer">
<div id='blankarea'>添加更多学校</div>
</div>
</div>
</div>
</div>
<script type="text/javascript">
$(document).ready(function () {
var clickElement = null;
$(".threepanels .ptreelist").bind("mousedown", function (event) {
//获取当前mousedown元素的内容
var itemContent = $(this).html();
var draggableDiv = $("#draggableDiv");
$(draggableDiv).css({ "display": "block", "height": 0 });
//将点击的元素内容复制
clickElement = $(this).clone();
var currentdiv = $(this).offset();
$(draggableDiv).css({ "top": currentdiv.top, "left": currentdiv.left });
draggableDiv.trigger(event);
//取消默认行为
return false;
});
$("#draggableDiv").mouseup(function (event) {
$(this).css({ "height": "0" });
});
//拖动元素时鼠标的位置
var dragDivLeft = 0;
var dragDivTop = 0;
$("#draggableDiv").draggable({
containment: "parent",
drag: function (event, ui) {
$("#draggableDiv").css({ "width": "260px", "height": "22px" });
$("#draggableDiv").append(clickElement);
var closeTop = $(".closeBar").offset().top;
dragDivLeft = event.target.offsetLeft;
dragDivTop = event.target.offsetTop;
},
stop: function () {
//拖拽结束,将拖拽容器内容清空
$("#draggableDiv").html("");
$("#draggableDiv").css({ "height": "0" });
}
});
//“放”的操作代码
$("#rightlistcontainer").droppable({
drop: function (event, ui) {
var childNode = $("#rightlistcontainer");
//数组记录右侧元素的上边和下边的坐标
var targetHeights = new Array();
var singleItemHeight = {}; //定义一个json对象
//".remove"就是一个区域的className
var childUlLength = $(event.target).find(".remove").length;
//只有一个remove区域块,无需循环计算
//如果右侧目前一个元素区域都没有
if ($("#rightlistcontainer").find(".remove").eq(0).length == 0) {
singleItemHeight["startHeight"] = 0;
singleItemHeight["endHeight"] = 0;
targetHeights.push(singleItemHeight);
}
else {
for (var i = 0; i < childUlLength; i++) {
singleItemHeight = {};//清空对象
var oUl = childNode.find(".remove").eq(i);
singleItemHeight["startHeight"] = parseInt(oUl.offset().top);
singleItemHeight["endHeight"] = parseInt(oUl.offset().top) + parseInt(oUl.outerHeight());
targetHeights.push(singleItemHeight);
}
}
var arrLength = targetHeights.length;
//标识在区域内,还是并排的区域
var isFlag = false;
for (var j = 0; j < arrLength; j++) {
//判断拖动的元素可以放到哪个区域中
if (dragDivTop > targetHeights[j].startHeight && dragDivTop < targetHeights[j].endHeight) {
var targetParent = $("#rightlistcontainer").find(".remove").eq(j);
var oSpan = "<span>或</span>";
targetParent.append(oSpan);
targetParent.append($("#draggableDiv").html());
isFlag = true;
}
}
if (!isFlag) {
//操作符html代码片段
var selecthtml = "<select style='margin: 5px 0;' name='itemrelation'><option>且</option><option>排除</option></select>";
//the first category drag into the panel, it will not show the select element.
//如果不是第一个单独区域块,就添加操作符
if ($("#rightlistcontainer").find(".remove").length != 0) {
$("#blankarea").before(selecthtml);
}
//区域块的div
var oDiv = $("<div class='remove'></div>");
var oUl = $("<ul class='ptreelist'></ul>")
//把draggableDiv中的内容值插入到目标div中
oDiv.append($("#draggableDiv").html());
$("#blankarea").before(oDiv);
}
}
});
});
</script>
</body>
</html>
你可以参考下下面这个网站 里面比较详细的介绍了如何使用jQuery Draggable和Droppable实现拖拽功能
http://www.poluoluo.com/jzxy/201307/232665.html
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>jQuery UI Draggable and Droppable Demo</title>
<style type="text/css">
/*reset style*/
body {
color: #333;
font-size: 14px;
line-height: 22px;
font-family: "Helvetica","Arial","微软雅黑","宋体";
}
ul, li, p {
padding: 0;
margin: 0;
}
a {
color: #0064a0;
padding: 1px;
text-decoration: none;
}
a:hover {
color: #26a0da;
text-decoration: underline;
}
#leftpanel {
float: left;
}
.peopletabs li {
list-style-type: none;
}
#maincontainer {
width: 800px;
height: 350px;
margin: 0 auto;
border: 2px solid #0069a0;
padding: 10px;
}
#schoolproperty {
height: 250px;
border: 1px solid green;
padding: 10px;
overflow: auto;
width: 350px;
float: left;
}
#selectedschool {
float: right;
}
#rightlistcontainer {
width: 300px;
height: 250px;
overflow: auto;
float: right;
border: 1px solid green;
padding: 10px;
}
#peopleproperty ul {
list-style-type: none;
}
.ptreelist li {
display: block;
width: 200px;
}
/*拖动的元素最好设置一个宽带和高度*/
#draggableDiv {
z-index: 1000;
background-color: Green;
width: 308px;
height: 22px;
position: absolute;
color: #fff;
}
#middlepanel {
width: 100px;
float: left;
margin-top: 120px;
}
#rightlistcontainer .remove {
padding: 5px;
border: 1px solid #b4b4b4;
background: #cdcdcd;
}
#rightlistcontainer .remove li {
width: 240px;
background-color: #cdcdcd;
padding: 0 5px;
background: #fff;
margin-top: 3px;
cursor: move;
}
#blankarea {
margin-top:10px;
height: 50px;
border: 1px dotted red;
}
</style>
<script src="http://code.jquery.com/jquery-1.9.1.js"></script>
<script src="http://code.jquery.com/ui/1.10.3/jquery-ui.js"></script>
</head>
<body>
<div id="maincontainer">
<div id='draggableDiv' class="ui-widget-content">
</div>
<span class="closeBar" style="float: right"><a href="return false;" title="关闭">X</a></span>
<div id="contentcontainer">
<!--左边面板-->
<div id="leftpanel">
<ul class="peopletabs">
<li><a class="current" href="#" panel-id="schoolproperty">高校</a></li>
<!--<li><a href="#" panel-id="behavior_list">Tab2</a></li>
<li><a href="#" panel-id="productintent_list">Tab3</a></li>-->
</ul>
<div id="schoolproperty" class="threepanels">
<ul class="ptreelist">
<li id="44">江西高校<span class="closebutton"></span>
<ul class="ptreelist">
<li id="4407">江西科技师范学院<span class="closebutton"></span></li>
</ul>
<ul class="ptreelist">
<li id="4408">江西科技学院<span class="closebutton"></span></li>
</ul>
</li>
</ul>
</div>
</div>
<div id="middlepanel">
<p>可以从左侧拖动条件到右侧</p>
</div>
<!--右边面板-->
<div id="selectedschool">
<p>
符合条件的学生数<span id="peopleaccount" class="red" style="font-size: 20px">1000</span>人
</p>
<div id="rightlistcontainer">
<div id='blankarea'>添加更多学校</div>
</div>
</div>
</div>
</div>
<script type="text/javascript">
$(document).ready(function () {
var clickElement = null;
$(".threepanels .ptreelist").bind("mousedown", function (event) {
//获取当前mousedown元素的内容
var itemContent = $(this).html();
var draggableDiv = $("#draggableDiv");
$(draggableDiv).css({ "display": "block", "height": 0 });
//将点击的元素内容复制
clickElement = $(this).clone();
var currentdiv = $(this).offset();
$(draggableDiv).css({ "top": currentdiv.top, "left": currentdiv.left });
draggableDiv.trigger(event);
//取消默认行为
return false;
});
$("#draggableDiv").mouseup(function (event) {
$(this).css({ "height": "0" });
});
//拖动元素时鼠标的位置
var dragDivLeft = 0;
var dragDivTop = 0;
$("#draggableDiv").draggable({
containment: "parent",
drag: function (event, ui) {
$("#draggableDiv").css({ "width": "260px", "height": "22px" });
$("#draggableDiv").append(clickElement);
var closeTop = $(".closeBar").offset().top;
dragDivLeft = event.target.offsetLeft;
dragDivTop = event.target.offsetTop;
},
stop: function () {
//拖拽结束,将拖拽容器内容清空
$("#draggableDiv").html("");
$("#draggableDiv").css({ "height": "0" });
}
});
//“放”的操作代码
$("#rightlistcontainer").droppable({
drop: function (event, ui) {
var childNode = $("#rightlistcontainer");
//数组记录右侧元素的上边和下边的坐标
var targetHeights = new Array();
var singleItemHeight = {}; //定义一个json对象
//".remove"就是一个区域的className
var childUlLength = $(event.target).find(".remove").length;
//只有一个remove区域块,无需循环计算
//如果右侧目前一个元素区域都没有
if ($("#rightlistcontainer").find(".remove").eq(0).length == 0) {
singleItemHeight["startHeight"] = 0;
singleItemHeight["endHeight"] = 0;
targetHeights.push(singleItemHeight);
}
else {
for (var i = 0; i < childUlLength; i++) {
singleItemHeight = {};//清空对象
var oUl = childNode.find(".remove").eq(i);
singleItemHeight["startHeight"] = parseInt(oUl.offset().top);
singleItemHeight["endHeight"] = parseInt(oUl.offset().top) + parseInt(oUl.outerHeight());
targetHeights.push(singleItemHeight);
}
}
var arrLength = targetHeights.length;
//标识在区域内,还是并排的区域
var isFlag = false;
for (var j = 0; j < arrLength; j++) {
//判断拖动的元素可以放到哪个区域中
if (dragDivTop > targetHeights[j].startHeight && dragDivTop < targetHeights[j].endHeight) {
var targetParent = $("#rightlistcontainer").find(".remove").eq(j);
var oSpan = "<span>或</span>";
targetParent.append(oSpan);
targetParent.append($("#draggableDiv").html());
isFlag = true;
}
}
if (!isFlag) {
//操作符html代码片段
var selecthtml = "<select style='margin: 5px 0;' name='itemrelation'><option>且</option><option>排除</option></select>";
//the first category drag into the panel, it will not show the select element.
//如果不是第一个单独区域块,就添加操作符
if ($("#rightlistcontainer").find(".remove").length != 0) {
$("#blankarea").before(selecthtml);
}
//区域块的div
var oDiv = $("<div class='remove'></div>");
var oUl = $("<ul class='ptreelist'></ul>")
//把draggableDiv中的内容值插入到目标div中
oDiv.append($("#draggableDiv").html());
$("#blankarea").before(oDiv);
}
}
});
});
</script>
</body>
</html>
你可以参考下下面这个网站 里面比较详细的介绍了如何使用jQuery Draggable和Droppable实现拖拽功能
http://www.poluoluo.com/jzxy/201307/232665.html
推荐律师服务:
若未解决您的问题,请您详细描述您的问题,通过百度律临进行免费专业咨询