
3个回答
展开全部
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace PrintBill
{
class Program
{
int ItemId;
int Amount;
int nongfushanquan = 0;
int jinmailang = 0;
int shuanghuihuotuichang = 0;
string s = string.Empty;
private void business()
{
Console.Write("输入商品编号:");
ItemId = Convert.ToInt32(Console.ReadLine());
Console.Write("输入商品数量:");
Amount = Convert.ToInt32(Console.ReadLine());
if (ItemId == 1)
{
nongfushanquan = nongfushanquan + Amount;
}
if (ItemId == 2)
{
jinmailang = jinmailang + Amount;
}
if (ItemId == 3)
{
shuanghuihuotuichang = shuanghuihuotuichang + Amount;
}
Console.WriteLine("输入e停止购物,输入其他任意键继续购物:");
s = Console.ReadLine();
}
private void ProduceBill()
{
double sum1=0;
double sum2=0;
double sum3=0;
Console.WriteLine("----------------欢迎光临光辉超市----------------");
Console.WriteLine("商品名称 单价 数量 小计");
if (nongfushanquan != 0)
{
sum1=nongfushanquan*1.02;
Console.WriteLine("农夫山泉 1.02" +" " + nongfushanquan + " " +"¥"+sum1);
}
if (jinmailang != 0)
{
sum2 = jinmailang * 3.5;
Console.WriteLine("今麦郎碗面 3.50" + " " + jinmailang + " " + "¥" + sum2);
}
if (shuanghuihuotuichang != 0)
{
sum3 = shuanghuihuotuichang * 0.5;
Console.WriteLine("双汇火腿肠 0.50" + " " + shuanghuihuotuichang + " " + "¥" + sum3);
}
double totalSum = sum1 + sum2 + sum3;
Console.WriteLine("总计:¥"+totalSum);
}
static void Main(string[] args)
{
Console.WriteLine("编号 商品名称");
Console.WriteLine("1 农夫山泉");
Console.WriteLine("2 今麦郎碗面");
Console.WriteLine("3 双汇火腿肠");
Console.WriteLine(" ");
Program p = new Program();
p.business();
while (p.s != "e")
{
p.business();
}
p.ProduceBill();
}
}
}
运行结果:
建议你自己再写写。
展开全部
//输出宽度自己调下吧
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Data.OleDb;
using System.Data;
namespace test
{
public class Goods
{
int number;
string name;
double price;
int buyCount;
public int Number
{
get { return this.number; }
set { this.number = value; }
}
public string Name
{
get { return this.name; }
set { this.name = value; }
}
public double Price
{
get { return this.price; }
set { this.price = value; }
}
public int BuyCount
{
get { return this.buyCount; }
set { this.buyCount = value; }
}
}
class Program
{
static void Main(string[] args)
{
Dictionary<int, string> initGoods = new Dictionary<int, string>();
initGoods.Add(1, "1;农夫山泉;2.50");
initGoods.Add(2, "2;今麦郎碗面;1.50");
initGoods.Add(3, "3;双汇火腿肠;1.00");
Dictionary<int, Goods> goodList = init(initGoods);//初始化商品
Console.WriteLine("编号 商品名称");
foreach (int item in goodList.Keys)
{
Console.Write(item);
Console.Write(" " + goodList[item].Name);
Console.WriteLine();
}
Console.WriteLine();
Dictionary<int, Goods> buyGoods = new Dictionary<int, Goods>();//购买商品
label1:
Console.Write("输入商品编号:");
int bianhao = Convert.ToInt32(Console.ReadLine());
if (!goodList.Keys.Contains(bianhao))
{
Console.WriteLine("不存在商品编号!");
Console.WriteLine();
goto label1;
}
if (!buyGoods.Keys.Contains(bianhao))
{
buyGoods.Add(bianhao, goodList[bianhao]);
}
Console.Write("输入购买数量:");
int buyGoodCount = Convert.ToInt32(Console.ReadLine());
buyGoods[bianhao].BuyCount += buyGoodCount;
Console.Write("输入e停止购物,输入其他任意键继续购物:");
string s = Console.ReadLine();
if (s.ToUpper() == "E")
{
goto print;
}
else
{
goto label1;
}
print:
Console.WriteLine();
Console.WriteLine("----------------欢迎光临光辉超市----------------");
Console.WriteLine("商品名称 单价 数量 小计");
foreach (int item in buyGoods.Keys)
{
Console.Write(buyGoods[item].Name);
Console.Write(" "+buyGoods[item].Price);
Console.Write(" " + buyGoods[item].BuyCount);
Console.Write(" ¥" + (buyGoods[item].Price * buyGoods[item].BuyCount));
Console.WriteLine();
}
Console.ReadKey();
}
private static Dictionary<int, Goods> init(Dictionary<int, string> goodsAndPrice)
{
Dictionary<int, Goods> goods = new Dictionary<int, Goods>();
Goods goodsSigle;
for (int i = 1; i <= goodsAndPrice.Count; i++)
{
goodsSigle = new Goods();
string[] temp = goodsAndPrice[i].ToString().Split(';');
goodsSigle.Number = Convert.ToInt32(temp[0]);
goodsSigle.Name = temp[1];
goodsSigle.Price = Convert.ToDouble(temp[2]);
goods.Add(goodsSigle.Number, goodsSigle);
}
return goods;
}
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Data.OleDb;
using System.Data;
namespace test
{
public class Goods
{
int number;
string name;
double price;
int buyCount;
public int Number
{
get { return this.number; }
set { this.number = value; }
}
public string Name
{
get { return this.name; }
set { this.name = value; }
}
public double Price
{
get { return this.price; }
set { this.price = value; }
}
public int BuyCount
{
get { return this.buyCount; }
set { this.buyCount = value; }
}
}
class Program
{
static void Main(string[] args)
{
Dictionary<int, string> initGoods = new Dictionary<int, string>();
initGoods.Add(1, "1;农夫山泉;2.50");
initGoods.Add(2, "2;今麦郎碗面;1.50");
initGoods.Add(3, "3;双汇火腿肠;1.00");
Dictionary<int, Goods> goodList = init(initGoods);//初始化商品
Console.WriteLine("编号 商品名称");
foreach (int item in goodList.Keys)
{
Console.Write(item);
Console.Write(" " + goodList[item].Name);
Console.WriteLine();
}
Console.WriteLine();
Dictionary<int, Goods> buyGoods = new Dictionary<int, Goods>();//购买商品
label1:
Console.Write("输入商品编号:");
int bianhao = Convert.ToInt32(Console.ReadLine());
if (!goodList.Keys.Contains(bianhao))
{
Console.WriteLine("不存在商品编号!");
Console.WriteLine();
goto label1;
}
if (!buyGoods.Keys.Contains(bianhao))
{
buyGoods.Add(bianhao, goodList[bianhao]);
}
Console.Write("输入购买数量:");
int buyGoodCount = Convert.ToInt32(Console.ReadLine());
buyGoods[bianhao].BuyCount += buyGoodCount;
Console.Write("输入e停止购物,输入其他任意键继续购物:");
string s = Console.ReadLine();
if (s.ToUpper() == "E")
{
goto print;
}
else
{
goto label1;
}
print:
Console.WriteLine();
Console.WriteLine("----------------欢迎光临光辉超市----------------");
Console.WriteLine("商品名称 单价 数量 小计");
foreach (int item in buyGoods.Keys)
{
Console.Write(buyGoods[item].Name);
Console.Write(" "+buyGoods[item].Price);
Console.Write(" " + buyGoods[item].BuyCount);
Console.Write(" ¥" + (buyGoods[item].Price * buyGoods[item].BuyCount));
Console.WriteLine();
}
Console.ReadKey();
}
private static Dictionary<int, Goods> init(Dictionary<int, string> goodsAndPrice)
{
Dictionary<int, Goods> goods = new Dictionary<int, Goods>();
Goods goodsSigle;
for (int i = 1; i <= goodsAndPrice.Count; i++)
{
goodsSigle = new Goods();
string[] temp = goodsAndPrice[i].ToString().Split(';');
goodsSigle.Number = Convert.ToInt32(temp[0]);
goodsSigle.Name = temp[1];
goodsSigle.Price = Convert.ToDouble(temp[2]);
goods.Add(goodsSigle.Number, goodsSigle);
}
return goods;
}
}
}
本回答被提问者采纳
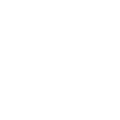
你对这个回答的评价是?
展开全部
这个其实还是很简单的,如果没有要求使用数据库,这个你就先用集合保存数据存在内存中
已赞过
已踩过<
评论
收起
你对这个回答的评价是?
推荐律师服务:
若未解决您的问题,请您详细描述您的问题,通过百度律临进行免费专业咨询