
一道java题 请设计一个学生类Student。属性包括:学号、姓名、英语成绩、数学成绩、计算机
一道java题请设计一个学生类Student。属性包括:学号、姓名、英语成绩、数学成绩、计算机成绩、总成绩。方法包括:构造方法、compare方法(比较两个学生的总成绩,...
一道java题
请设计一个学生类Student。属性包括:学号、姓名、英语成绩、数学成绩、计算机成绩、总成绩。方法包括:构造方法、compare方法(比较两个学生的总成绩,结果分大于、小于、等于),sum方法(计算总成绩)、testScore方法(计算评测成绩,可以取三门课成绩的平均分)。
老师布置的作业,求求各位大神了! 展开
请设计一个学生类Student。属性包括:学号、姓名、英语成绩、数学成绩、计算机成绩、总成绩。方法包括:构造方法、compare方法(比较两个学生的总成绩,结果分大于、小于、等于),sum方法(计算总成绩)、testScore方法(计算评测成绩,可以取三门课成绩的平均分)。
老师布置的作业,求求各位大神了! 展开
7个回答
2015-06-22
展开全部
public class Student
{
private String stuId;
private String name;
private float englishScore;
private float mathScore;
private float computerScore;
private float sumScore;
public Student()
{
}
public Student(String stuId, String name, float englishScore,
float mathScore, float computerScore)
{
this.stuId = stuId;
this.name = name;
this.englishScore = englishScore;
this.mathScore = mathScore;
this.computerScore = computerScore;
this.sumScore = sum();
}
public String getStuId()
{
return stuId;
}
public void setStuId(String stuId)
{
this.stuId = stuId;
}
public String getName()
{
return name;
}
public void setName(String name)
{
this.name = name;
}
public float sum()
{
return sumScore = englishScore + mathScore + computerScore;
}
public float testScore()
{
return sum()/3;
}
public String compare(Student student)
{
if(this.sumScore > student.sum())
return "大于";
else if(this.sumScore == student.sum())
return "等于";
else return "小于";
}
public static void main(String[] args)
{
Student tim = new Student("0001", "tim", 90, 90, 90);
Student tom = new Student("0001", "tom", 80, 90, 95);
System.out.println("tim的测评成绩:"+tim.testScore());
System.out.println("tom的测评成绩:"+tom.testScore());
System.out.println("tim总成绩 "+tim.compare(tom)+" tom总成绩");
}
}
上面的代码作为作业应该够用了,如果应用最好重写equals和hashCo方法。
更多追问追答
追问
谢谢你*^_^*
追答
不客气。
展开全部
ublic class Student { // 学号 private String studentNo; // 姓名 private String name; // 英语成绩 private Double enlishScore; // 数学成绩 private Double mathScore; // 计算机 private Computer computer; public String getStudentNo() { return studentNo; } public void setStudentNo(String studentNo) { this.studentNo = studentNo; } public String getName() { return name; } public void setName(String name) { this.name = name; } public Double getEnlishScore() { return enlishScore; } public void setEnlishScore(Double enlishScore) { this.enlishScore = enlishScore; } public Double getMathScore() { return mathScore; } public void setMathScore(Double mathScore) { this.mathScore = mathScore; } public Computer getComputer() { return computer; } public void setComputer(Computer computer) { this.computer = computer; }} /** * 计算机类 * * @author Administrator * */class Computer { // 计算机名 private String name; // 系统版本号 private String sysInfo; public String getName() { return name; } public void setName(String name) { this.name = name; } public String getSysInfo() { return sysInfo; } public void setSysInfo(String sysInfo) { this.sysInfo = sysInfo; } }
已赞过
已踩过<
评论
收起
你对这个回答的评价是?
展开全部
public class Student implements Comparable<Student> {
private int id;
private String name;
private int englishScore;
private int mathScore;
private int computerScore;
private int totalScore;
public Student(int id, String name, int englishScore, int mathScore,
int computerScore, int totalScore) {
this.id = id;
this.name = name;
this.englishScore = englishScore;
this.mathScore = mathScore;
this.computerScore = computerScore;
this.totalScore = sum();
}
public int sum() {
return englishScore + mathScore + computerScore;
}
public int compareTo(Student s) {
if(this.totalScore > s.totalScore) {
return -1;
} else if(this.totalScore == s.totalScore) {
return 0;
} else {
return 1;
}
}
public double testScore() {
return (double)this.totalScore/3;
}
}
更多追问追答
追问
谢谢谢谢!
我可以加你吗?我还有几道题(^_^)
本回答被提问者采纳
已赞过
已踩过<
评论
收起
你对这个回答的评价是?
展开全部
public class Student {
// 学号
private String studentNo;
// 姓名
private String name;
// 英语成绩
private Double enlishScore;
// 数学成绩
private Double mathScore;
// 计算机
private Computer computer;
public String getStudentNo() {
return studentNo;
}
public void setStudentNo(String studentNo) {
this.studentNo = studentNo;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Double getEnlishScore() {
return enlishScore;
}
public void setEnlishScore(Double enlishScore) {
this.enlishScore = enlishScore;
}
public Double getMathScore() {
return mathScore;
}
public void setMathScore(Double mathScore) {
this.mathScore = mathScore;
}
public Computer getComputer() {
return computer;
}
public void setComputer(Computer computer) {
this.computer = computer;
}
}
/**
* 计算机类
*
* @author Administrator
*
*/
class Computer {
// 计算机名
private String name;
// 系统版本号
private String sysInfo;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getSysInfo() {
return sysInfo;
}
public void setSysInfo(String sysInfo) {
this.sysInfo = sysInfo;
}
}
已赞过
已踩过<
评论
收起
你对这个回答的评价是?
2016-12-20 · 企业短信服务平台。
关注
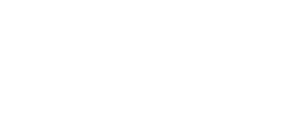
展开全部
package com.zhidao20161213;
public class Student {
private String num;
private String name;
private double math;
private double english;
private double computer;
public String getNum() {
return num;
}
public void setNum(String num) {
this.num = num;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getMath() {
return math;
}
public void setMath(double math) {
this.math = math;
}
public double getEnglish() {
return english;
}
public void setEnglish(double english) {
this.english = english;
}
public double getComputer() {
return computer;
}
public void setComputer(double computer) {
this.computer = computer;
}
}
已赞过
已踩过<
评论
收起
你对这个回答的评价是?
推荐律师服务:
若未解决您的问题,请您详细描述您的问题,通过百度律临进行免费专业咨询