
C++中如何比较两个字符变量的大小 5
4个回答
展开全部
C++提供的字符串处理函数在string.h的头文件中声明。
字符串比较函数strcmp( )
函数原型:int strcmp(const char *s1, const char *s2);
int strncmp(const char *s1, const char *s2, int n);
功能:以字典顺序方式比较两个字符串是否相等。如果两个串相等,函数返回值为0;如果s1串大于s2串,返回值大于0;如果s1串小于s2串,返回值小于0。函数strcmp用于对两个串的完全比较;函数strncmp用于比较两个串的前n个字符。
【例5- 6】字符串比较。
#include <iostream.h>
#include <string.h>
#include <iomanip.h>
void main()
{
char s1[] = "Happy New Year";
char s2[] = "Happy New Year";
char s3[] = "Happy Holidays";
cout << "s1 = " << s1 << "\ns2 = "<< s2
<< "\ns3 = "<< s3 << "\n\nstrcmp(s1, s2) ="
<< setw( 2 ) << strcmp( s1, s2 )
<< "\nstrcmp(s1, s3) = "<< setw( 2 )
<< strcmp( s1, s3 ) << "\nstrcmp(s3, s1) ="
<< setw( 2 ) << strcmp( s3, s1 );
cout << "\n\nstrncmp(s1, s3, 6) = "<< setw( 2 )
<< strncmp( s1, s3, 6) << "\nstrncmp(sl, s3, 7) ="
<< setw( 2 ) << strncmp( s1, s3, 7 )
<< "\nstrncmp(s3, s1, 7) ="
<< setw( 2 ) << strncmp( s3, s1, 7 ) << endl;
}
程序运行结果如下:
s1 = Happy New Year
s2 = Happy New Year
s3 = Happy Holidays
strcmp(s1, s2) = 0
strcmp(s1, s3) = 1
strcmp(s3, s1) = -1
strncmp(s1, s3, 6) = 0
strncmp(s1, s3, 7) = 1
strncmp(s3, s1, 7) = -1
自定义版字符串比较
//当str1>str2时,返回正数
//当str1==str2时,返回0
//当str1<str2时,返回负数
int strcmp(const char *str1,const char *str2)
{
int x;
while(*str1!='\0'||*str2!='\0' )
{
if(*str1==*str2)
{
x=0;
str1++;
str2++;
continue;
}
if(*str1>*str2)
{ x=1;break ;}
if(*str1<*str2)
{ x=-1;break; }
if(*str1=='\0'&&*str2!='\0' )
{
x=-1; break;
}
if(*str1!='\0'&&*str2=='\0' )
{
x=1; break;
}
if(*str1=='\0' ||*str2=='\0' )
{
x=0;continue;
}
}
return x;
}
#include<iostream.h>
void main()
{
char*str1="Happy New Year!";
char*str2="Happy New Year!";
char*str3="Happy Holidays!";
cout<<"str1="<<str1<<"\nstr2="<<str2<<"\nstr3="<<str3<<endl;
cout<<"strcmp(str1,str2)="<<strcmp(str1,str2)
<<"\nstrcmp(str1,str3)="<<strcmp(str1,str3)
<<"\nstrcmp(str3,str1)="<<strcmp(str3,str1)<<endl;
}
字符串比较函数strcmp( )
函数原型:int strcmp(const char *s1, const char *s2);
int strncmp(const char *s1, const char *s2, int n);
功能:以字典顺序方式比较两个字符串是否相等。如果两个串相等,函数返回值为0;如果s1串大于s2串,返回值大于0;如果s1串小于s2串,返回值小于0。函数strcmp用于对两个串的完全比较;函数strncmp用于比较两个串的前n个字符。
【例5- 6】字符串比较。
#include <iostream.h>
#include <string.h>
#include <iomanip.h>
void main()
{
char s1[] = "Happy New Year";
char s2[] = "Happy New Year";
char s3[] = "Happy Holidays";
cout << "s1 = " << s1 << "\ns2 = "<< s2
<< "\ns3 = "<< s3 << "\n\nstrcmp(s1, s2) ="
<< setw( 2 ) << strcmp( s1, s2 )
<< "\nstrcmp(s1, s3) = "<< setw( 2 )
<< strcmp( s1, s3 ) << "\nstrcmp(s3, s1) ="
<< setw( 2 ) << strcmp( s3, s1 );
cout << "\n\nstrncmp(s1, s3, 6) = "<< setw( 2 )
<< strncmp( s1, s3, 6) << "\nstrncmp(sl, s3, 7) ="
<< setw( 2 ) << strncmp( s1, s3, 7 )
<< "\nstrncmp(s3, s1, 7) ="
<< setw( 2 ) << strncmp( s3, s1, 7 ) << endl;
}
程序运行结果如下:
s1 = Happy New Year
s2 = Happy New Year
s3 = Happy Holidays
strcmp(s1, s2) = 0
strcmp(s1, s3) = 1
strcmp(s3, s1) = -1
strncmp(s1, s3, 6) = 0
strncmp(s1, s3, 7) = 1
strncmp(s3, s1, 7) = -1
自定义版字符串比较
//当str1>str2时,返回正数
//当str1==str2时,返回0
//当str1<str2时,返回负数
int strcmp(const char *str1,const char *str2)
{
int x;
while(*str1!='\0'||*str2!='\0' )
{
if(*str1==*str2)
{
x=0;
str1++;
str2++;
continue;
}
if(*str1>*str2)
{ x=1;break ;}
if(*str1<*str2)
{ x=-1;break; }
if(*str1=='\0'&&*str2!='\0' )
{
x=-1; break;
}
if(*str1!='\0'&&*str2=='\0' )
{
x=1; break;
}
if(*str1=='\0' ||*str2=='\0' )
{
x=0;continue;
}
}
return x;
}
#include<iostream.h>
void main()
{
char*str1="Happy New Year!";
char*str2="Happy New Year!";
char*str3="Happy Holidays!";
cout<<"str1="<<str1<<"\nstr2="<<str2<<"\nstr3="<<str3<<endl;
cout<<"strcmp(str1,str2)="<<strcmp(str1,str2)
<<"\nstrcmp(str1,str3)="<<strcmp(str1,str3)
<<"\nstrcmp(str3,str1)="<<strcmp(str3,str1)<<endl;
}
展开全部
这两个看似一样的东西,其实是指向不同的地址,所以他们比较的时候在比较的是指针所指向的地址,当然会不同。
而且你写错了:
const char* x;
x = "3.1415926";
string y = "3.1415926";
if(x.c_str()==y)
return 1;
这个x是const char*类型,怎么可能能.c_str()呢。。。应该是if(x==y.c_str())吧。
正确的比较方法:
const char* x;
x = "3.1415926";
string y = "3.1415926";
if(*x==*y.c_str())
return 1;
使用*把他们都从指针变回非指针类型即可。
而且你写错了:
const char* x;
x = "3.1415926";
string y = "3.1415926";
if(x.c_str()==y)
return 1;
这个x是const char*类型,怎么可能能.c_str()呢。。。应该是if(x==y.c_str())吧。
正确的比较方法:
const char* x;
x = "3.1415926";
string y = "3.1415926";
if(*x==*y.c_str())
return 1;
使用*把他们都从指针变回非指针类型即可。
已赞过
已踩过<
评论
收起
你对这个回答的评价是?
展开全部
字符的大小,可以直接用< > ==等逻辑运算符。
比如 char a = '1';
char b = '2';
if(b>a)cout<<"b>a"<<endl;
else cout<<"a>=b"<<endl;
比如 char a = '1';
char b = '2';
if(b>a)cout<<"b>a"<<endl;
else cout<<"a>=b"<<endl;
本回答被网友采纳
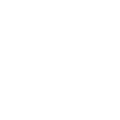
你对这个回答的评价是?
展开全部
string类直接用比较运算符 <,>,=
已赞过
已踩过<
评论
收起
你对这个回答的评价是?
推荐律师服务:
若未解决您的问题,请您详细描述您的问题,通过百度律临进行免费专业咨询