
android 圈中activity中的部分对象并获得这些对象
比如一个activity中有几个对象(圆、矩形、三角形、椭圆),然后在屏幕上圈出其中部分对象,并获得圈内的对象。这个该怎么实现呢?或者可以说我用ontouchevent画...
比如一个activity中有几个对象(圆、矩形、三角形、椭圆),然后在屏幕上圈出其中部分对象,并获得圈内的对象。
这个该怎么实现呢?
或者可以说我用ontouchevent画个圈以后,怎么判断这些对象是否存在于画的圈里面 展开
这个该怎么实现呢?
或者可以说我用ontouchevent画个圈以后,怎么判断这些对象是否存在于画的圈里面 展开
1个回答
推荐于2016-03-03 · 知道合伙人教育行家
关注
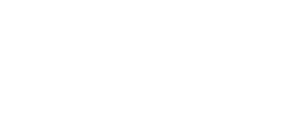
展开全部
可以查看这两篇文章:
1,通过Serializable传递一个类对象的例子
http://mingkg21.iteye.com/blog/438913
2,通过Parcelable传递一个类对象的例子
http://mingkg21.iteye.com/blog/463895
而若需要传递多个类对象的时候就必须用Parcelable来封装类,然后将其存放在ArrayList,
我从网上下载的一个例子该成这种情况的:可以看其代码:
对象:
Java代码
package cn.wizhy;
import android.os.Parcel;
import android.os.Parcelable;
public class Phone implements Parcelable{
String type;
String company;
int price;
public Phone(String t,String c,int p) {
type=t;
company=c;
price=p;
}
public Phone() {
// TODO Auto-generated constructor stub
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public String getCompany() {
return company;
}
public void setCompany(String company) {
this.company = company;
}
public int getPrice() {
return price;
}
public void setPrice(int price) {
this.price = price;
}
public static final Parcelable.Creator<Phone> CREATOR = new Creator<Phone>(){
@Override
public Phone createFromParcel(Parcel source) {
// TODO Auto-generated method stub
Phone cus = new Phone();
cus.type = source.readString();
cus.company = source.readString();
cus.price = source.readInt();
return cus;
}
@Override
public Phone[] newArray(int size) {
// TODO Auto-generated method stub
return new Phone[size];
}
};
@Override
public int describeContents() {
// TODO Auto-generated method stub
return 0;
}
@Override
public void writeToParcel(Parcel dest, int flags) {
// TODO Auto-generated method stub
dest.writeString(type);
dest.writeString(company);
dest.writeInt(price);
}
}
第一个Activity,构造类将其存放到Arraylist里,并通过Intent传给第二个Activity
Java代码
package cn.wizhy;
import java.util.ArrayList;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
public class Demo extends Activity {
ArrayList<Phone> info = new ArrayList<Phone>();
public Phone phone;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
insertPhone();
Intent intent = new Intent(this,Demo2.class);
// Bundle bundle = new Bundle();
// bundle.putSerializable("phone", phone);
// intent.putExtras(bundle);
phone = new Phone("goole","G1",6000);
info.add(phone);
phone = new Phone("apple", "iphone3G", 5000);
info.add(phone);
intent.putExtra("phones", info);
startActivity(intent);
}
public void insertPhone(){
phone= new Phone("apple", "iphone3G", 5000);
}
}
第二个Activity接受数据:
Java代码
package cn.wizhy;
import java.util.ArrayList;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
public class Demo2 extends Activity {
ArrayList<Phone> info = new ArrayList<Phone>();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
Intent intent = getIntent();
info =intent.getParcelableArrayListExtra("phones");
for(int i=0;i<info.size();i++){
System.out.println("type="+info.get(i).type+" company="+info.get(i).company+" price"+info.get(i).price);
}
}
}
http://aijiawang-126-com.iteye.com/blog/643762
1,通过Serializable传递一个类对象的例子
http://mingkg21.iteye.com/blog/438913
2,通过Parcelable传递一个类对象的例子
http://mingkg21.iteye.com/blog/463895
而若需要传递多个类对象的时候就必须用Parcelable来封装类,然后将其存放在ArrayList,
我从网上下载的一个例子该成这种情况的:可以看其代码:
对象:
Java代码
package cn.wizhy;
import android.os.Parcel;
import android.os.Parcelable;
public class Phone implements Parcelable{
String type;
String company;
int price;
public Phone(String t,String c,int p) {
type=t;
company=c;
price=p;
}
public Phone() {
// TODO Auto-generated constructor stub
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public String getCompany() {
return company;
}
public void setCompany(String company) {
this.company = company;
}
public int getPrice() {
return price;
}
public void setPrice(int price) {
this.price = price;
}
public static final Parcelable.Creator<Phone> CREATOR = new Creator<Phone>(){
@Override
public Phone createFromParcel(Parcel source) {
// TODO Auto-generated method stub
Phone cus = new Phone();
cus.type = source.readString();
cus.company = source.readString();
cus.price = source.readInt();
return cus;
}
@Override
public Phone[] newArray(int size) {
// TODO Auto-generated method stub
return new Phone[size];
}
};
@Override
public int describeContents() {
// TODO Auto-generated method stub
return 0;
}
@Override
public void writeToParcel(Parcel dest, int flags) {
// TODO Auto-generated method stub
dest.writeString(type);
dest.writeString(company);
dest.writeInt(price);
}
}
第一个Activity,构造类将其存放到Arraylist里,并通过Intent传给第二个Activity
Java代码
package cn.wizhy;
import java.util.ArrayList;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
public class Demo extends Activity {
ArrayList<Phone> info = new ArrayList<Phone>();
public Phone phone;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
insertPhone();
Intent intent = new Intent(this,Demo2.class);
// Bundle bundle = new Bundle();
// bundle.putSerializable("phone", phone);
// intent.putExtras(bundle);
phone = new Phone("goole","G1",6000);
info.add(phone);
phone = new Phone("apple", "iphone3G", 5000);
info.add(phone);
intent.putExtra("phones", info);
startActivity(intent);
}
public void insertPhone(){
phone= new Phone("apple", "iphone3G", 5000);
}
}
第二个Activity接受数据:
Java代码
package cn.wizhy;
import java.util.ArrayList;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
public class Demo2 extends Activity {
ArrayList<Phone> info = new ArrayList<Phone>();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
Intent intent = getIntent();
info =intent.getParcelableArrayListExtra("phones");
for(int i=0;i<info.size();i++){
System.out.println("type="+info.get(i).type+" company="+info.get(i).company+" price"+info.get(i).price);
}
}
}
http://aijiawang-126-com.iteye.com/blog/643762
追问
不是传对象。。。是看圈中了哪些对象
追答
哦
本回答被提问者和网友采纳
已赞过
已踩过<
评论
收起
你对这个回答的评价是?
推荐律师服务:
若未解决您的问题,请您详细描述您的问题,通过百度律临进行免费专业咨询