
JAVA 如何获取并替换XML格式的字符串
<b><id>456</id></b>"
把b-id节点的值456修改为789
使结果为strnew=="<a><id>123</id></a>
<b><id>789</id></b>" 展开
2018-07-12 · 国内最优秀java资源共享平台
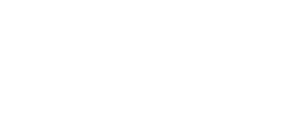
xml文件:
<?xml version="1.0" encoding="UTF-8"?>
<transaction>
<body>
<request>
<tranTyp>批量业务现存</tranTyp>
<acctNm>0085213560</acctNm>
<acctNo>6225885517843413</acctNo>
<avlBal>201958.65</avlBal>
<acctTyp>0</acctTyp>
<tranTime>20170801101030</tranTime>
<currencyTyp>CNY</currencyTyp>
<tranDesc></tranDesc>
<bal>201958.65</bal>
<tranAmt>100000.00</tranAmt>
</request>
</body>
<header>
<msg>
<sndTm>101019</sndTm>
<msgCd>WCS0000200</msgCd>
<seqNb>632376531000009</seqNb>
<sndMbrCd>5200</sndMbrCd>
<rcvMbrCd>0000</rcvMbrCd>
<sndDt>20170821</sndDt>
<sndAppCd>CBS</sndAppCd>
<rcvAppCd>WCS</rcvAppCd>
<callTyp>SYN</callTyp>
</msg>
<ver>1.0</ver>
<pnt>
<sndTm>101216</sndTm>
<sndMbrCd>0000</sndMbrCd>
<rcvMbrCd>0000</rcvMbrCd>
<sndDt>20170809</sndDt>
<sndAppCd>ESB</sndAppCd>
<rcvAppCd>WCS</rcvAppCd>
</pnt>
</header>
</transaction>
2. java实现实例:
public class Test {
/**
*
* @param document
* Document对象(读xml生成的)
* @return String字符串
* @throws Throwable
*/
public String xmlToString(Document document) throws Throwable {
TransformerFactory ft = TransformerFactory.newInstance();
Transformer ff = ft.newTransformer();
ff.setOutputProperty("encoding", "GB2312");
ByteArrayOutputStream bos = new ByteArrayOutputStream();
ff.transform(new DOMSource(document), new StreamResult(bos));
return bos.toString();
}
/**
*
* @param xml形状的str串
* @return Document 对象
*/
public Document StringTOXml(String str) {
StringBuilder sXML = new StringBuilder();
sXML.append(str);
DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance();
Document doc = null;
try {
InputStream is = new ByteArrayInputStream(sXML.toString().getBytes("utf-8"));
doc = dbf.newDocumentBuilder().parse(is);
is.close();
} catch (Exception e) {
e.printStackTrace();
}
return doc;
}
/**
*
* @param document
* @return 某个节点的值 前提是需要知道xml格式,知道需要取的节点相对根节点所在位置
*/
public String getNodeValue(Document document, String nodePaht) {
XPathFactory xpfactory = XPathFactory.newInstance();
XPath path = xpfactory.newXPath();
String servInitrBrch = "";
try {
servInitrBrch = path.evaluate(nodePaht, document);
} catch (XPathExpressionException e) {
e.printStackTrace();
}
return servInitrBrch;
}
/**
*
* @param document
* @param nodePath
* 需要修改的节点相对根节点所在位置
* @param vodeValue
* 替换的值
*/
public void setNodeValue(Document document, String nodePath, String vodeValue) {
XPathFactory xpfactory = XPathFactory.newInstance();
XPath path = xpfactory.newXPath();
Node node = null;
;
try {
node = (Node) path.evaluate(nodePath, document, XPathConstants.NODE);
} catch (XPathExpressionException e) {
e.printStackTrace();
}
node.setTextContent(vodeValue);
}
3. 测试
public static void main(String[] args) throws Throwable {
// 读取xml文件,生成document对象
DocumentBuilder builder = DocumentBuilderFactory.newInstance().newDocumentBuilder();
// 文件的位置在工作空间的根目录(位置随意,只要写对就ok)
Document document = builder.parse(new File("a.xml"));
Test t = new Test();
// XML————》String
String str = t.xmlToString(document);
System.out.println("str:" + str);
// String ————》XML
Document doc = t.StringTOXml(str);
String nodePath = "/transaction/header/msg/sndMbrCd";
// getNodeValue
String nodeValue = t.getNodeValue(doc, nodePath);
System.out.println("修改前nodeValue:" + nodeValue);
// setNodeValue
t.setNodeValue(doc, nodePath, nodeValue + "hello");
System.out.println("修改后nodeValue:" + t.getNodeValue(doc, nodePath));
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
测试结果:
str:<?xml version="1.0" encoding="UTF-8" standalone="no"?><transaction>
<body>
<request>
<tranTyp>批量业务现存</tranTyp>
<acctNm>0085213560</acctNm>
<acctNo>6225885517843413</acctNo>
<avlBal>201958.65</avlBal>
<acctTyp>0</acctTyp>
<tranTime>20170801101030</tranTime>
<currencyTyp>CNY</currencyTyp>
<tranDesc/>
<bal>201958.65</bal>
<tranAmt>100000.00</tranAmt>
</request>
</body>
<header>
<msg>
<sndTm>101019</sndTm>
<msgCd>WCS0000200</msgCd>
<seqNb>632376531000009</seqNb>
<sndMbrCd>5200</sndMbrCd>
<rcvMbrCd>0000</rcvMbrCd>
<sndDt>20170821</sndDt>
<sndAppCd>CBS</sndAppCd>
<rcvAppCd>WCS</rcvAppCd>
<callTyp>SYN</callTyp>
</msg>
<ver>1.0</ver>
<pnt>
<sndTm>101216</sndTm>
<sndMbrCd>0000</sndMbrCd>
<rcvMbrCd>0000</rcvMbrCd>
<sndDt>20170809</sndDt>
<sndAppCd>ESB</sndAppCd>
<rcvAppCd>WCS</rcvAppCd>
</pnt>
</header>
</transaction>结果显示
修改前nodeValue:5200
修改后nodeValue:5200hello