
在ASP.NET中如何调用 oracle 存储过程调用
如下的存储过程,在ASP.NET中如何调用createorreplaceprocedurexiaoming.test_product(productIDinnumber,...
如下的存储过程,在ASP.NET中如何调用
create or replace procedure xiaoming.test_product
(
productID in number,
OutCursor out sys_refcursor
)
as
Begin
open OutCursor for
select * from xiaoming.product
where productid=productID;
End; 展开
create or replace procedure xiaoming.test_product
(
productID in number,
OutCursor out sys_refcursor
)
as
Begin
open OutCursor for
select * from xiaoming.product
where productid=productID;
End; 展开
3个回答
展开全部
调用存储过程方法:
//存储过程
//proName 存储过程名称
//values 存储过程参数
public int ExecutePro(string proName,SqlParameter[]values)
{
SqlCommand cmd = new SqlCommand();
cmd.Connection = con;
cmd.CommandText = proName;
cmd.Parameters.AddRange(values);
cmd.CommandType = CommandType.StoredProcedure;
try
{
con.Open();
cmd.ExecuteNonQuery();
return int.Parse(values[values.Length - 1].Value.ToString());
}
catch
{
return -1;
}
finally
{
con.Close();
}
}
例如:
#region 注册用户
/// <summary>
/// 注册用户
/// </summary>
/// <param name="user">用户对象</param>
/// <returns>
/// 0 成功
///-1 失败
///1 已存在
///</returns>
public static int Regist(UserInfo user)
{
//procedure pro_Regist(@ub_loginId varchar(50),@up_pwd text,@ud_Name varchar(20),@ud_sex bit,@ud_phone char(11),@ud_remark varchar(50),@flag int output)
SqlParameter[] param = {
new SqlParameter("@ub_loginId",user.U_ub_loginid),
new SqlParameter("@up_pwd",FormsAuthentication.HashPasswordForStoringInConfigFile( user.U_ub_pwd,"MD5")),
new SqlParameter("@ud_Name",user.U_ud_Name),
new SqlParameter("@ud_sex",user.U_ud_sex),
new SqlParameter("@ud_phone",user.U_ud_phone),
new SqlParameter("@ud_remark",user.U_ud_remark),
new SqlParameter("@flag",0)
};
param[param.Length-1].Direction = ParameterDirection.Output;
return DBHelper.CreateInstance().ExecutePro("pro_Regist", param);
}
#endregion
//存储过程
//proName 存储过程名称
//values 存储过程参数
public int ExecutePro(string proName,SqlParameter[]values)
{
SqlCommand cmd = new SqlCommand();
cmd.Connection = con;
cmd.CommandText = proName;
cmd.Parameters.AddRange(values);
cmd.CommandType = CommandType.StoredProcedure;
try
{
con.Open();
cmd.ExecuteNonQuery();
return int.Parse(values[values.Length - 1].Value.ToString());
}
catch
{
return -1;
}
finally
{
con.Close();
}
}
例如:
#region 注册用户
/// <summary>
/// 注册用户
/// </summary>
/// <param name="user">用户对象</param>
/// <returns>
/// 0 成功
///-1 失败
///1 已存在
///</returns>
public static int Regist(UserInfo user)
{
//procedure pro_Regist(@ub_loginId varchar(50),@up_pwd text,@ud_Name varchar(20),@ud_sex bit,@ud_phone char(11),@ud_remark varchar(50),@flag int output)
SqlParameter[] param = {
new SqlParameter("@ub_loginId",user.U_ub_loginid),
new SqlParameter("@up_pwd",FormsAuthentication.HashPasswordForStoringInConfigFile( user.U_ub_pwd,"MD5")),
new SqlParameter("@ud_Name",user.U_ud_Name),
new SqlParameter("@ud_sex",user.U_ud_sex),
new SqlParameter("@ud_phone",user.U_ud_phone),
new SqlParameter("@ud_remark",user.U_ud_remark),
new SqlParameter("@flag",0)
};
param[param.Length-1].Direction = ParameterDirection.Output;
return DBHelper.CreateInstance().ExecutePro("pro_Regist", param);
}
#endregion
2012-07-11 · 知道合伙人软件行家
关注
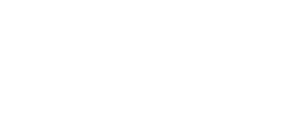
展开全部
(1)执行一个没有参数的存储过程的代码如下:
SqlConnection conn=new SqlConnection(“connectionString”);
SqlDataAdapter da = new SqlDataAdapter();
da.SelectCommand = new SqlCommand();
da.SelectCommand.Connection = conn;
da.SelectCommand.CommandText = "NameOfProcedure";
da.SelectCommand.CommandType = CommandType.StoredProcedure;
然后只要选择适当的方式执行此处过程,用于不同的目的即可。
(2)执行一个有参数的存储过程的代码如下(我们可以将调用存储过程的函数声明为ExeProcedure(string inputdate)):
SqlConnection conn=new SqlConnection(“connectionString”);
SqlDataAdapter da = new SqlDataAdapter();
da.SelectCommand = new SqlCommand();
da.SelectCommand.Connection = conn;
da.SelectCommand.CommandText = "NameOfProcedure";
da.SelectCommand.CommandType = CommandType.StoredProcedure;
(以上代码相同,以下为要添加的代码)
param = new SqlParameter("@ParameterName", SqlDbType.DateTime);
param.Direction = ParameterDirection.Input;
param.Value = Convert.ToDateTime(inputdate);
da.SelectCommand.Parameters.Add(param);
这样就添加了一个输入参数。若需要添加输出参数:
param = new SqlParameter("@ParameterName", SqlDbType.DateTime);
param.Direction = ParameterDirection.Output;
param.Value = Convert.ToDateTime(inputdate);
da.SelectCommand.Parameters.Add(param);
若要获得参储过程的返回值:
param = new SqlParameter("@ParameterName", SqlDbType.DateTime);
param.Direction = ParameterDirection.ReturnValue;
param.Value = Convert.ToDateTime(inputdate);
da.SelectCommand.Parameters.Add(param);
SqlConnection conn=new SqlConnection(“connectionString”);
SqlDataAdapter da = new SqlDataAdapter();
da.SelectCommand = new SqlCommand();
da.SelectCommand.Connection = conn;
da.SelectCommand.CommandText = "NameOfProcedure";
da.SelectCommand.CommandType = CommandType.StoredProcedure;
然后只要选择适当的方式执行此处过程,用于不同的目的即可。
(2)执行一个有参数的存储过程的代码如下(我们可以将调用存储过程的函数声明为ExeProcedure(string inputdate)):
SqlConnection conn=new SqlConnection(“connectionString”);
SqlDataAdapter da = new SqlDataAdapter();
da.SelectCommand = new SqlCommand();
da.SelectCommand.Connection = conn;
da.SelectCommand.CommandText = "NameOfProcedure";
da.SelectCommand.CommandType = CommandType.StoredProcedure;
(以上代码相同,以下为要添加的代码)
param = new SqlParameter("@ParameterName", SqlDbType.DateTime);
param.Direction = ParameterDirection.Input;
param.Value = Convert.ToDateTime(inputdate);
da.SelectCommand.Parameters.Add(param);
这样就添加了一个输入参数。若需要添加输出参数:
param = new SqlParameter("@ParameterName", SqlDbType.DateTime);
param.Direction = ParameterDirection.Output;
param.Value = Convert.ToDateTime(inputdate);
da.SelectCommand.Parameters.Add(param);
若要获得参储过程的返回值:
param = new SqlParameter("@ParameterName", SqlDbType.DateTime);
param.Direction = ParameterDirection.ReturnValue;
param.Value = Convert.ToDateTime(inputdate);
da.SelectCommand.Parameters.Add(param);
追问
我返回的是一个游标,游标返回的内容是一个数据集。这样如何获取返回值???
本回答被网友采纳
已赞过
已踩过<
评论
收起
你对这个回答的评价是?
展开全部
正解 如下
已赞过
已踩过<
评论
收起
你对这个回答的评价是?
推荐律师服务:
若未解决您的问题,请您详细描述您的问题,通过百度律临进行免费专业咨询