
如何终止Android Handler 中的消息处理
1个回答
2015-03-28 · 知道合伙人数码行家
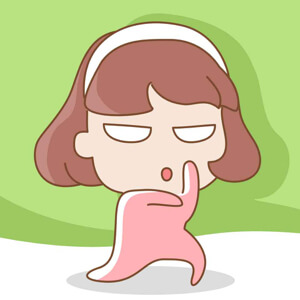
知道合伙人数码行家
采纳数:117538
获赞数:517165
长期从事计算机组装,维护,网络组建及管理。对计算机硬件、操作系统安装、典型网络设备具有详细认知。
向TA提问 私信TA
关注
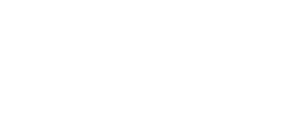
展开全部
在Android中,activity做为主线程,如其他线程需要与其交互,要在message队列中进行处理。至于Handler、Message、MessageQueue、looper在网上都有很多详细说明,讲的也就是将消息或线程通过handler放入消息队列,looper用于消息队列中就行消息间的通信,在消息队列的尾部,通过Handler来取出消息进行处理,即handlermessage()方法,采用的是先进先出的方式处理消息.
下面是自己写的一个小例子:
1.public class HandlerTest1 extends Activity {
2. /** Called when the activity is first created. */
3. private TextView text = null;
4. private Button but1 = null;
5. private Button but2 = null;
6. @Override
7. public void onCreate(Bundle savedInstanceState) {
8. super.onCreate(savedInstanceState);
9. setContentView(R.layout.main);
10. text = (TextView) findViewById(R.id.text);
11. but1 = (Button) findViewById(R.id.but1);
12. but2 = (Button) findViewById(R.id.but2);
13. but1.setOnClickListener(new OnClickListener(){
14.
15. @Override
16. public void onClick(View v) {
17. // TODO Auto-generated method stub
18. handler.post(r); //将线程加入到消息队列中
19. }
20.
21. });
22. but2.setOnClickListener(new OnClickListener(){
23.
24. @Override
25. public void onClick(View v) {
26. // TODO Auto-generated method stub
27. handler.removeCallbacks(r); //清空消息队列
28. }
29.
30. });
31. }
32. Handler handler = new Handler(){
33.
34. @Override
35. public void handleMessage(Message msg) {
36. // TODO Auto-generated method stub
37. text.setText(String.valueOf(msg.arg1));
38. System.out.println(msg.arg1);
39. handler.postDelayed(r, 2000); //将线程重新加入到消息队列中,产生一种循环
40.
41. }
42.
43. };
44.
45. Runnable r = new Runnable(){
46. int i = 0;
47. @Override
48. public void run() {
49. // TODO Auto-generated method stub
50. System.out.println("runnable");
51. Message msg = handler.obtainMessage(); //获得message对象
52. msg.arg1=i; //arg1,arg2 ,bunder,obj等作为消息传递数据
53. i++;
54. handler.sendMessage(msg); //发送消息
55. }
56.
57. };
58.
59.
60.}
1.<?xml version="1.0" encoding="utf-8"?>
2.<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
3. android:orientation="vertical"
4. android:layout_width="fill_parent"
5. android:layout_height="fill_parent"
6. >
7.<TextView
8. android:id="@+id/text"
9. android:layout_width="fill_parent"
10. android:layout_height="wrap_content"
11. android:text="hello"
12. />
13.<Button
14. android:id="@+id/but1"
15. android:layout_width="fill_parent"
16. android:layout_height="wrap_content"
17. android:text="开始"
18. />
19.<Button
20. android:id="@+id/but2"
21. android:layout_width="fill_parent"
22. android:layout_height="wrap_content"
23. android:text="结束"
24. />
25.</LinearLayout>
本篇文章来源于 Linux公社网站(www.linuxidc.com) 原文链接:http://www.linuxidc.com/Linux/2011-08/40054.htm
下面是自己写的一个小例子:
1.public class HandlerTest1 extends Activity {
2. /** Called when the activity is first created. */
3. private TextView text = null;
4. private Button but1 = null;
5. private Button but2 = null;
6. @Override
7. public void onCreate(Bundle savedInstanceState) {
8. super.onCreate(savedInstanceState);
9. setContentView(R.layout.main);
10. text = (TextView) findViewById(R.id.text);
11. but1 = (Button) findViewById(R.id.but1);
12. but2 = (Button) findViewById(R.id.but2);
13. but1.setOnClickListener(new OnClickListener(){
14.
15. @Override
16. public void onClick(View v) {
17. // TODO Auto-generated method stub
18. handler.post(r); //将线程加入到消息队列中
19. }
20.
21. });
22. but2.setOnClickListener(new OnClickListener(){
23.
24. @Override
25. public void onClick(View v) {
26. // TODO Auto-generated method stub
27. handler.removeCallbacks(r); //清空消息队列
28. }
29.
30. });
31. }
32. Handler handler = new Handler(){
33.
34. @Override
35. public void handleMessage(Message msg) {
36. // TODO Auto-generated method stub
37. text.setText(String.valueOf(msg.arg1));
38. System.out.println(msg.arg1);
39. handler.postDelayed(r, 2000); //将线程重新加入到消息队列中,产生一种循环
40.
41. }
42.
43. };
44.
45. Runnable r = new Runnable(){
46. int i = 0;
47. @Override
48. public void run() {
49. // TODO Auto-generated method stub
50. System.out.println("runnable");
51. Message msg = handler.obtainMessage(); //获得message对象
52. msg.arg1=i; //arg1,arg2 ,bunder,obj等作为消息传递数据
53. i++;
54. handler.sendMessage(msg); //发送消息
55. }
56.
57. };
58.
59.
60.}
1.<?xml version="1.0" encoding="utf-8"?>
2.<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
3. android:orientation="vertical"
4. android:layout_width="fill_parent"
5. android:layout_height="fill_parent"
6. >
7.<TextView
8. android:id="@+id/text"
9. android:layout_width="fill_parent"
10. android:layout_height="wrap_content"
11. android:text="hello"
12. />
13.<Button
14. android:id="@+id/but1"
15. android:layout_width="fill_parent"
16. android:layout_height="wrap_content"
17. android:text="开始"
18. />
19.<Button
20. android:id="@+id/but2"
21. android:layout_width="fill_parent"
22. android:layout_height="wrap_content"
23. android:text="结束"
24. />
25.</LinearLayout>
本篇文章来源于 Linux公社网站(www.linuxidc.com) 原文链接:http://www.linuxidc.com/Linux/2011-08/40054.htm
已赞过
已踩过<
评论
收起
你对这个回答的评价是?
推荐律师服务:
若未解决您的问题,请您详细描述您的问题,通过百度律临进行免费专业咨询