
C++编程题:编写一个函数,求一个字符串的长度,在main函数中输入字符串,并输出其长度。 20
#include<stdio.h>
#include<stdlib.h>
#define N 1024
int Strlen(char* str) {
int count = 0;
while (*str != '\0') {
count += 1;
++str;
}
return count;
}
void main() {
char str[N];
char* p = str;
printf("请输入str\n");
for (int i = 0, ch = '\0';ch != '\n'; ++i) {
ch = getchar();
if (i >= N) {//当超出字符串长度时, 继续接受键盘输入的字符,直到输入\n为止
/这是为了防止字符串输入完成后输入的字符会被下一个需要输入的数据接收
continue;
}
if (ch == '\n' || i == N - 1) {
p[i] = '\0';
continue;
}
p[i] = ch;
}
printf("这个字符串为:%s\n", p);
printf("这个字符串的长度为:%d\n", Strlen(p));
system("pause");
}
运行效果:
扩展资料:
include用法:
#include命令预处理命令的一种,预处理命令可以将别的源代码内容插入到所指定的位置;可以标识出只有在特定条件下才会被编译的某一段程序代码;可以定义类似标识符功能的宏,在编译时,预处理器会用别的文本取代该宏。
插入头文件的内容
#include命令告诉预处理器将指定头文件的内容插入到预处理器命令的相应位置。有两种方式可以指定插入头文件:
1、#include<文件名>
2、#include"文件名"

2024-07-18 广告
int i = 1;
while (*ch++ != '\0') //字符串用指针指向, 字符串末尾肯定是\0 所以指到\0的时候结束循环
{
i++; //每循环一次 长度就+1。
}
#include <iostream>
#include <string>
using namespace std;
int changdu(string s)
{
int size;
size=s.length();
return size;
}
int main ()
{
int chang;
string str;
cin>>str;
chang=changdu(str);
cout << "The size of str is " << chang << " characters.\n";
return 0;
}
编得比较难看。。。
还是可以用的
输出的 字符串长度 不对吧
如果 我输入字符串123 那应该是 4 不是 3把 。。
刚才 我又 把 你的 改了一下 就运行正确了
能帮忙加个注释么//。。。比如为什么int size 还有int changdu 还有string什么意思。。
你多输入了一个空格吧,那么就把cin>>str;换成getline(cin,str);
#include <stdio.h>
#include <string.h>//使用strlen需要此头文件
int my_strlen(char* str)
{
char *p = str;
int count = 0;
while(*(p++) != '\0')
count++;
return count;
}
int main()
{
char str[1024]={0};
printf("输入您的字符串:\n");
scanf("%s", str);
printf("系统函数获得的字符串长度:%d\n", strlen(str));
printf("自定义获得字符串函数:%d\n", my_strlen(str));
return 0;
}
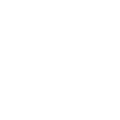
#include <string>
using namespace std;
int strLength(const string strCont)
{
const char* pStr = strCont.data();
string::size_type index = 0;
while(*pStr != '\0')
{
++index;
++pStr;
}
return index;
}
int main(void)
{
string strCont;
cin>>strCont;
strLength(strCont);
system("pause");
return 0;
}
没看到 输出的 长度 。。
没加输出语句,在strLength(strCont)后面再加上cout<<strLength(strCont)<<endl;即可输出结果,你再试下。