
c++编写一个点类Point,功能包括输出点的坐标,移动到新位置及输出它与另一个点的距离 5
主程序如下:
void main()
{
Point a,b,c;
a.set(7.8,9.8);
b.set(34.5,67.8);
c=a;
cout<<"a和b两点之距为:"<<a.Distance(b)<<endl;
cout<<"b和c两点之距为:"<<b.Distance(c)<<endl;
cout<<"a和c两点之距为:"<<a.Distance(c)<<endl;
c.move(26.7,58);
cout<<"c("<<c.getx()<<","<<c.gety()<<")"<<endl;
}
提示:用来输出两点距离的函数的原型为double Distance(Point&)
大神帮讲解一下,老师让预习,完全看不懂啊 展开
该类可以提供移动,求到另一点的距离,获取X坐标和Y坐标等操作,也可以设置X坐标和Y坐标的值。要求有拷贝构造函数。数据成员为X坐标和Y坐标。
源代码如下
using namespace std;
class Point{
public:
Point(); //不带参数构造函数
Point(int X, int Y); //带参数的构造函数
Point(const Point &p); //拷贝构造函数;
int getX(); //获取横坐标
int getY(); //获取纵坐标
void setX(int iX); //设置横坐标
void setY(int iY); //设置纵坐标
float distance(Point p1, Point p2); //计算两点的距离protected:
private:
int x; //横坐标
int y; //纵坐标
};
int main()
{
Point pos;
Point pt(10, 10);
Point pts(pt);
cout << "获取pos的坐标值" << endl
cout << "X:" << pos.getX() << endl;
cout << "Y:" << pos.getY() << endl;
pos.setX(20);
pos.setY(20);
cout << "获取pos的设置后的坐标值" << endl;
cout << "X:" << pos.getX() << endl;
cout << "Y:" << pos.getY() << endl;
cout << "获取pt的坐标值" << endl;
cout << "X:" << pt.getX() << endl;
cout << "Y:" << pt.getY() << endl;
cout << "获取pts的坐标值" << endl;
cout << "X:" << pos.getX() << endl;
cout << "Y:" << pos.getY() << endl;
cout << "输出pos与pt之间的距离" << endl;
cout << "X:" << pos.distance(pos, pt) << endl;
return 0;
}
扩展资料
一个点类Point,有2个私有数据成员x,y代表点坐标的源代码
include
#include
using namespace std;
class Point
{
public:
Point(int a,int b):x(a),y(b){cout<<"初始化点类的一个对象\n";}
~Point(){cout<<"回收点类的内存空间\n";}
void show()
{cout<<"这个点的坐标为:"<<"("< double distance(Point &p){return sqrt((p.y-y)
(p.x-y)+(p.x-x)*(p.x-x));}
private:
int x;
int y;
};
int main()
{
Point a(0,0);
Point b(1,1);
cout< return 0;
}
#include<iostream>
#include<cmath>
usingnamespacestd;
classPoint{//坐标点类
public:
constdoublex,y;
Point(doublex=0.0,doubley=0.0):x(x),y(y){}
doubledistanceTo(Pointp)const{//到指定点的距离
returnsqrt((x-p.x)*(x-p.x)+(y-p.y)*(y-p.y));
}
};
intmain(){
Pointa(1.0,8.0),b(5.0,2.0);//两个点,数据可以自己定
cout<<"距离是:"<<a.distanceTo(b)<<endl;//调用函数计算a和b之间的距离
return0;
}
扩展资料
设计一个点类Point,包括私有数据成员x和y,成员函数包括公有的构造函数和析构函数。再设计一个矩形类Rectangle,矩形类使用两个Point类的对象p1,p2作为矩形的对角顶点,具有公有的构造函数,以及可以输出2对坐标值和面积的Print函数。在main函数中定义Rectangle类的对象rect,调用Print函数输出其位置和面积。(类中可适当增加成员函数和缺省值)
#include<iostream>
usingnamespacestd;
classpoint
{
private:
intx,y;
public:
point(intx,inty):x(x),y(y)
{
}
intgetx(){returnx;}
intgety(){returny;}
~point()
{
}
};
classrectangle
{
private:
pointp1,p2;
public:
rectangle(intx1,inty1,intx2,inty2):p1(x1,y1),p2(x2,y2)
{
}
voidprint()
{
cout<<p1.getx()<<p1.gety()<<endl;
cout<<p2.getx()<<p2.gety()<<endl;
cout<<(p2.getx()-p1.getx())*(p2.gety()-p1.gety())<<endl;
}
};
voidmain()
{
rectanglerect(0,0,1,2);
rect.print();
}
#include <iostream>
#include <cmath>
using namespace std;
class Point
{
private:
double x;
double y;
public :
Point()
{
}
Point(double _x, double _y)
{
x = _x;
y = _y;
}
void set(double _x, double _y)
{
x = _x;
y = _y;
}
double getx()
{
return x;
}
double gety()
{
return y;
}
double Distance(Point p)
{
return sqrt((x - p.getx()) * (x - p.getx()) + (y - p.gety()) * (y - p.gety()));
}
void move(double _x, double _y)
{
x = x + _x;
y = y + _y;
}
};
void main()
{
Point a,b,c;
a.set(7.8,9.8);
b.set(34.5,67.8);
c=a;
cout<<"a和b两点之距为:"<<a.Distance(b)<<endl;
cout<<"b和c两点之距为:"<<b.Distance(c)<<endl;
cout<<"a和c两点之距为:"<<a.Distance(c)<<endl;
c.move(26.7,58);
cout<<"c("<<c.getx()<<","<<c.gety()<<")"<<endl;
}
设置a的坐标(7.8,9.8) b(34.5,67.8) c和a一样
输出ab的距离
输出bc的距离
输出ac的距离
move函数c点移动到(26.7,58)这点
然后输出c的坐标
private:
double x;
double y;
public:
Point();
Point(double x1, double y1) {x=x1;y=y1}
void set(double x1, double y1) {x=x1;y=y1}
void Display() {cout << "(x,y)=(" << x << "," << y << ")" << endl;
void move(double x1, double y1) {x=x1;y=y1}
double getx() {return x;}
double gety() { return y;}
double Distance(Point &p) {
return sqrt( pow((p.getx()-x), 2) + pow((p.gety()-y),2));
}
};
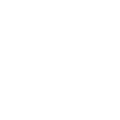