
c语言读取txt文档并将内容存放到表里
一、大概了解
c语言文件的操作分 读 和 写,读指的是:将文件内的信息写入到程序,可以理解为
硬盘信息->内存信息 的转化方式
而写指的是:将程序中的信息写入到文件,如txt文件。是一种
内存信息->硬盘信息 的转化方式
对于你的问题,则是 读 的方式,
二、具体使用方法
在C语言中,读文件的需要用到文件类型 FILE;
通常的使用方式为: FILE *fp;定义一个文件指针;
用指针打开文件的函数为:fp = fopen("mm.txt","w"));w可以是r,对应两种使用方式:w(write),r(read)
如果是将信息写入文件,则是w。
若是将文件信息写入程序,则是r。
在r的方式时,即读文件的方式时,若打开的文件不存在,则会打开失败。
在w的方式时。即在写进文件的,比如你讲信息写入“cc.mm”这么一个文件里时,程序发现并没“cc.mm”那么一个文件,则会自动创建一个新文件 命名为“cc.mm”并将信息写入其中。注意!后缀名 .xx、.tet、.什么都无所谓,都默认按txt的方式写入。
文件的读取函数为:fwrite(p, sizeof(XX), 1, fp); 即将文件内的信息,按XX大小的内存写入指针P当中。通过函数调用之后,fp即文件指针自动后移。
文件写入的函数为:fprintf(fp,"%x",p );即将p指针里的信息,按照%x的格式写入文件中。
在最后一定要记得使用fclose(fp)!!!!关闭文件指针
的时候我给你一段代码加深理解
#include <string.h>
#include <windows.h>
#define NEW (TX *)malloc(sizeof(TX))
typedef struct tongxunlu //定义的一个通讯录的结构体
{
char number[10]; //号码
char name[20]; //姓名
int old; //年龄
char address[20]; //地址
char phone[12]; //电话
struct tongxunlu *next;//链表
}TX;
int main ()
{
FILE *fp; // 文件指针fp
TX *p;
int i,n; // i,n控制循环
if((fp = fopen("学生信息.txt","w")) == NULL ) // 打开文件
{
printf(" 人品 不够!!启动失败!!!\n");
exit(0);
}
printf("输入人数:"); // 键盘输入人数n
scanf("%d",&n);
fprintf(fp,"%d",n); // 将人数n写入文件
fprintf(fp,"\n");
p = NEW; // 开辟一个新内存,并让指针p指向它
printf(" 输入档案号、姓名、年龄、籍贯、联系电话\n");
for(i = 0; n > i ; i++) // 反复用同一个节点进行n次循环
{
scanf("%s",&p -> number); // 键盘将数据写入该节点
scanf("%s",&p -> name);
scanf("%d",&p -> old);
scanf("%s",&p -> address);
scanf("%s",&p -> phone);
fprintf(fp,"%s ",p -> number); // 将该节点内的数据写入文件
fprintf(fp,"%s ",p -> name);
fprintf(fp,"%d ",p -> old);
fprintf(fp,"%s ",p -> address);
fprintf(fp,"%s ",p -> phone);
fprintf(fp,"\n");
}
free(p); // 释放p内存
fclose(fp); // 关闭文件
printf("\n\n数据输入完成\n");
}
2016-06-14 · 知道合伙人互联网行家
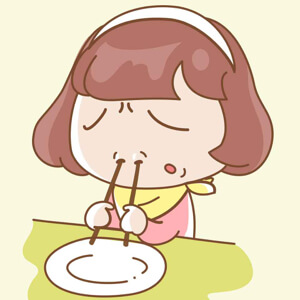
知道合伙人互联网行家
向TA提问 私信TA
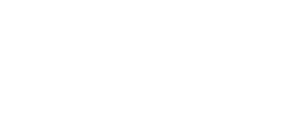
2.使用fscan()函数对文件进行读取。
3.放到二维数组其实就是读取相应格式的数据,然后对应二维数组的每个位置
例如
#include "stdio.h"
#define M 300
#define N 50
void main()
{
int i,j;
float a[M][N]={0};
FILE *fp;
if((fp=fopen("test.txt","rt"))==NULL)
{
printf("cannot open file\n");
return;
}
for(i=0;i<M;i++)
{
for(j=0;j<N;j++)
fscanf(fp,"%f",&a[i][j]);
fscanf(fp,"\n");
}
fclose(fp);
for(i=0;i<M;i++)
{
for(j=0;j<N;j++)
printf("%g ",a[i][j]);
printf("\n");
}
}
供大家参考:
1)
#include <stdio.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <stdlib.h>
#include <string.h>
#define SIZE 200
char *change[SIZE];
int num = 0;
void fun(char *p)
{
char *q;
while(*p == '[') {
change[num++] = ++p;
while(*p++!=']');
*(p-1) = '\0';
}
}
int main(void)
{
int fd,ret = 0;
int i;
char buf[SIZE];
fd = open("./abc.txt",O_RDWR);
if(ret $amp; perror("open fail");
exit(1);
}
ret = read(fd , buf , sizeof(buf));
printf("%s\n",buf);
fun(buf);
for(i = 0; i $amp; printf("%s\n",change[i]);
return 0;
}
2)
用fscanf的正则表达就可以了
#include <stdio.h>
int main(int argc, char* argv[])
{
FILE *fp1;
int i;
char other[20];
char a[5][20] = {0};
if((fp1 = fopen("abc.txt","r")) == NULL)
{
printf("open file error\n");
return -1;
}
for (i=0; i<5; i++)
{
fscanf(fp1, "%[^0-9a-zA-Z]s", &other);
if (fscanf(fp1, "%[0-9a-zA-Z]s", a[i]) == EOF )
{
break;
}
}
for (; i>0; i--)
{
printf("%d = %s\n", i-1, a[i-1]);
}
return 0;
}
输出
1 = bbbbb
0 = aaaaa
2.使用fscan()函数对文件进行读取。
3.放到二维数组其实就是读取相应格式的数据,然后对应二维数组的每个位置
例如
#include "stdio.h"
#define M 300
#define N 50
void main()
{
int i,j;
float a[M][N]={0};
FILE *fp;
if((fp=fopen("test.txt","rt"))==NULL)
{
printf("cannot open file\n");
return;
}
for(i=0;i<M;i++)
{
for(j=0;j<N;j++)
fscanf(fp,"%f",&a[i][j]);
fscanf(fp,"\n");
}
fclose(fp);
for(i=0;i<M;i++)
{
for(j=0;j<N;j++)
printf("%g ",a[i][j]);
printf("\n");
}
}
int main (void) {
FILE *fp = fopen("file.txt","w"); // 打开一个文件 以写入的方式打开
char arr[10] = "hello";
fseek (arr, 1,sizeof (arr),fp); // 向文件写入一个数据块
fclose (fp) // 关闭文件
}
记得采纳呀!!